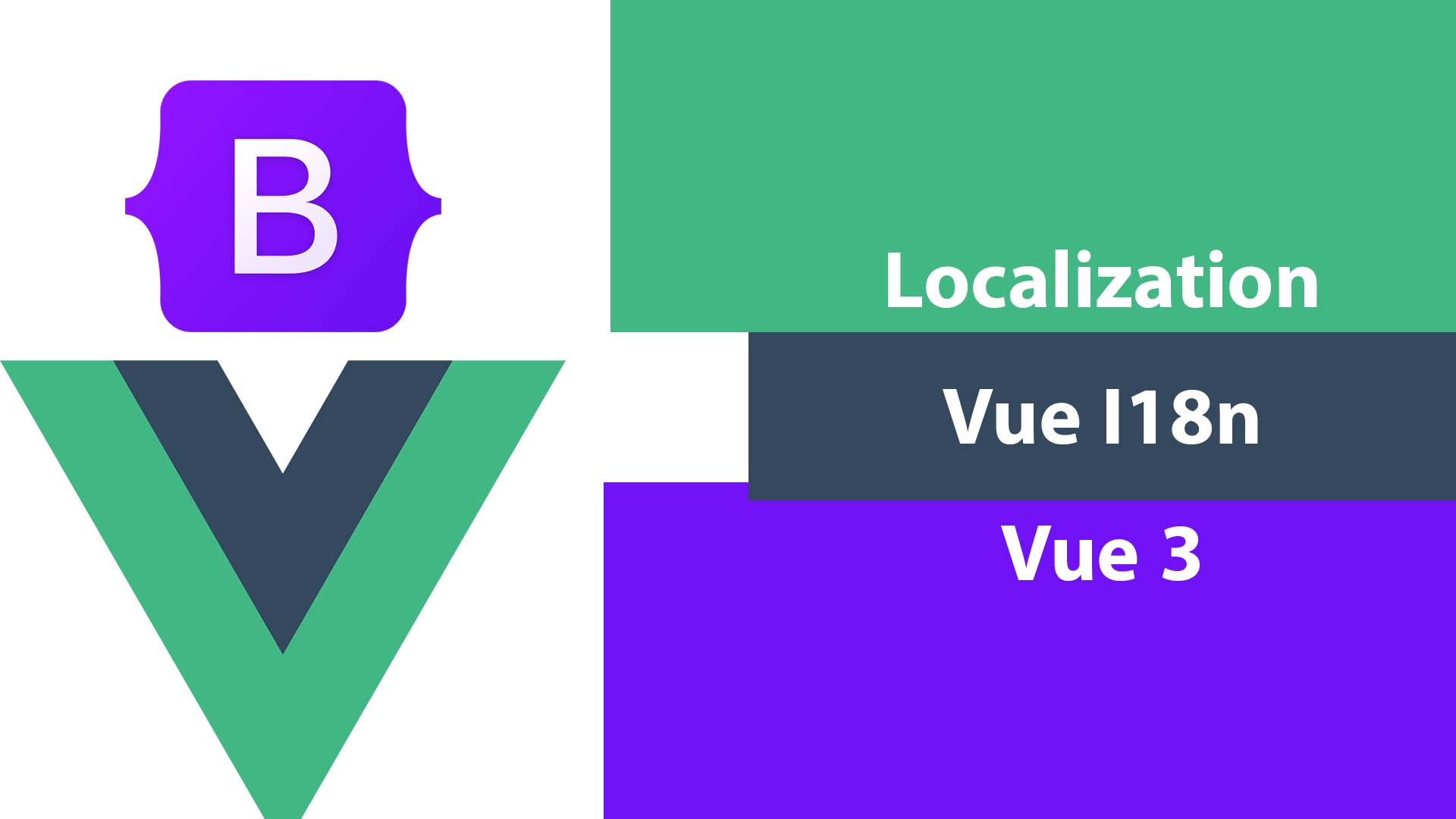
Let’s make a Vue 3 multi-language web application that allows the user to change the interface language.
If you live outside countries that speak English like me, you may know that Multi-language websites or applications are mandatory to learn. So let’s learn localization, We will create a navbar with a change website language option that changes the website direction from left to right to right to left depending on the current app language. And The website will support English and Arabic language.
Vue I18n
Let’s install Vue i18n, the internationalization plugin for Vue.js, this package removes the pain of localizing a website.
npm install vue-i18n@9
//or
yarn add vue-i18n@9
After installation, we will create a new file src\i18n.js
for configuration.
import { createI18n } from 'vue-i18n'
import en from './locales/en.json'
import ar from './locales/ar.json'
function loadLocaleMessages() {
const locales = [{ en: en }, { ar: ar }]
const messages = {}
locales.forEach(lang => {
const key = Object.keys(lang)
messages[key] = lang[key]
})
return messages
}
export default createI18n({
locale: 'en',
fallbackLocale: 'en',
messages: loadLocaleMessages()
})
We imported 2 files en.json and ar.json that we will create later, those files contain all the translations. The loadLocaleMessages function is for getting the translation key and value for vue-i18n
. The last thing is creating and configuring Vue I18n via createI18n
. We set the main language as “en” for English and the fallback language as “en” as well and finally we load the translation.
Let’s add i18n to our application src\main.js
import i18n from './i18n'
createApp(App).use(i18n).mount('#app')
Translations
We will create a JSON file for each language, for Arabic src\locales\ar.json
and English src\locales\en.json
. We need to remember that in every property the Key must be the same in all files and the value holds the translation
{
"Home" :"الرئيسية",
"About":"من نحن",
}
{
"Home" :"Home",
"About":"About Us",
}
Now you can use the translation in any component as {{$t('Home')}}
or {{$t('About')}}
Vue I18n Example
Let’s create a navbar with a change website language option and change the website direction from left to right to right to left
<template>
<nav class="navbar navbar-expand-lg navbar-dark bg-dark" :dir="$i18n.locale == 'ar'?'rtl':'ltr'">
<div class="container-fluid">
<router-link :to="{ name: 'Home' }" class="navbar-brand d-flex align-items-center">
<i class="bi bi-box2-heart h1 me-1"></i>
<strong>VUE</strong>
</router-link>
<button class="navbar-toggler" type="button" data-bs-toggle="collapse" data-bs-target="#navbarNav" aria-controls="navbarNav" aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="collapse navbar-collapse" id="navbarNav">
<ul class="navbar-nav">
<li class="nav-item me-3">
<router-link class="nav-link" :class="$route.name == 'Home'? 'active':''" aria-current="page" :to="{ name: 'Home' }">
{{$t('Home')}}
</router-link>
</li>
<li class="nav-item me-3">
<router-link class="nav-link" :class="$route.name == 'About'? 'active':''" aria-current="page" :to="{ name: 'About' }">
{{$t('About')}}
</router-link>
</li>
</ul>
<div class="locale-changer">
<select v-model="$i18n.locale">
<option v-for="locale in $i18n.availableLocales" :key="`locale-${locale}`" :value="locale">{{ locale }}</option>
</select>
</div>
</div>
</div>
</nav>
</template>
Let’s explain, at the <nav>
tag we used :dir="$i18n.locale == 'ar'?'rtl':'ltr'"
to change the navbar direction. We used $i18n.locale
to know the current application language.
We translate the navbar links {{$t(‘Home’)}} and {{$t(‘About’)}} by using the Keys only from the translation files.
Finally, we created a dropdown selection form input for changing the website language and it will do that automatically if we try to change the value of $i18n.locale
with one language form src\locales
.
That’s all, thank you