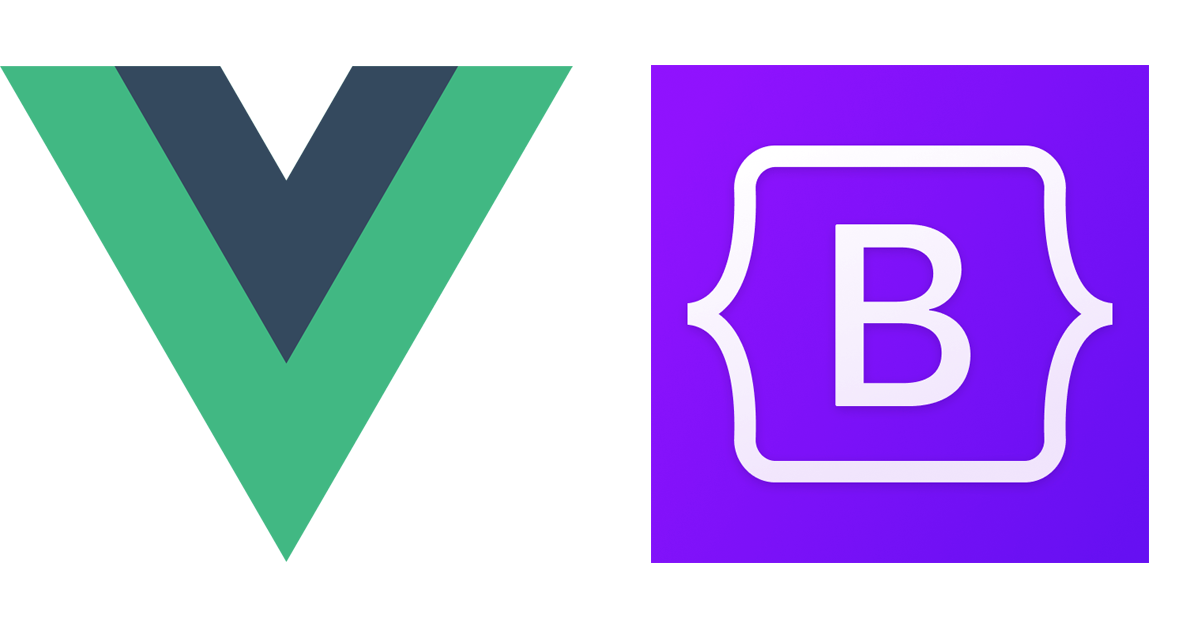
Using Bootstrap’s Modal inside your Vue 3 application is a little bit tricky but it is worth it, Bootstrap’s Modal uses JavaScript to add dialogs to your site for lightboxes, user notifications, or completely custom content.
We are not going to talk about using any libraries, I will demonstrate here the example by using pure bootstrap 5 with Vue 3 in the Vue CLI project.
Learn How to Create Vuejs Website with Vue CLI And Vue Router.
What will we do?
I will use the bootstrap’s modal as an alert so First, we create the main Vue component for the Modal. Second, we add the props for hiding and displaying the Modal and color variants. Finally creating the open/close function and the slot
for adding HTML text to the Modal.
Creating the modal component.
Inside the “components” folder, we create the “alerts” folder. the first Vue component for the modal will be “AlertDefault.vue” that will be the default, we can import it into other modal components for other purposes like show errors or info.
we create another file in the same folder “ErrorAlert.vue” for displaying error alert.
Adding codes to the modal component.
First, we will create the JS script code like the following:
<script>
export default {
name: 'AlertDefault',
props: {
visible: Boolean,
variant:String,
},
data(){
return{
OpenClose:this.visible
}
},
methods:{
OpenCloseFun(){
this.OpenClose =false;
},
},
watch: {
visible: function(newVal, oldVal) { // watch it
this.OpenClose =newVal;
console.log('new' +newVal+ '==' +oldVal)
}
}
}
</script>
Let’s explain the code:
props
: we havevisible
, the boolean to hide or show the modal andvariant
for adding boostrap color variant classes likedanger
,info
andsuccess
.data
:OpenClose
to take the value ofvisible
and using for the toggle method.methods
: OpenCloseFun is used for closing the modal.watch
: To watch any change on visible.
the code for the template will be as the following:
<template>
<div >
<!-- Modal -->
<div v-if="OpenClose" class="modal fade show"
tabindex="-1" aria-labelledby="exampleModalLabel" aria-modal="true" role="dialog"
style="display:block">
<div class="modal-dialog modal-dialog-centered">
<div class="modal-content">
<div class="modal-header">
<button type="button" @click="OpenCloseFun()" class="btn-close" ></button>
</div>
<div class="modal-body">
<slot></slot>
</div>
<div class="modal-footer">
<button type="button" @click="OpenCloseFun()" :class="'btn btn-'+variant" >{{$t('Close')}}</button>
</div>
</div>
</div>
</div>
</div>
</template>
Creating the “ErrorAlert.vue”
<template>
<AlertDefault variant="danger" :msg="msg" :visible="visible">
<p class="text-center"><svg xmlns="http://www.w3.org/2000/svg" width="64" height="64" fill="#dc3545" class="bi bi-exclamation-triangle-fill" viewBox="0 0 16 16">
<path d="M8.982 1.566a1.13 1.13 0 0 0-1.96 0L.165 13.233c-.457.778.091 1.767.98 1.767h13.713c.889 0 1.438-.99.98-1.767L8.982 1.566zM8 5c.535 0 .954.462.9.995l-.35 3.507a.552.552 0 0 1-1.1 0L7.1 5.995A.905.905 0 0 1 8 5zm.002 6a1 1 0 1 1 0 2 1 1 0 0 1 0-2z"/>
</svg></p>
<p class="text-center">{{msg}}</p>
</AlertDefault>
</template>
<script>
import AlertDefault from './AlertDefault.vue'
export default {
name: 'ErrorAlert',
components:{
AlertDefault
},
props: {
msg: String,
visible: Boolean,
},
}
</script>
Using the modal component.
You can import the “ErrorAlert” as you want inside your code and toggle visible
.
import ErrorAlert from '@/components/alerts/ErrorAlert.vue'
<ErrorAlert :msg="errorMgs" :visible="ShowError" />