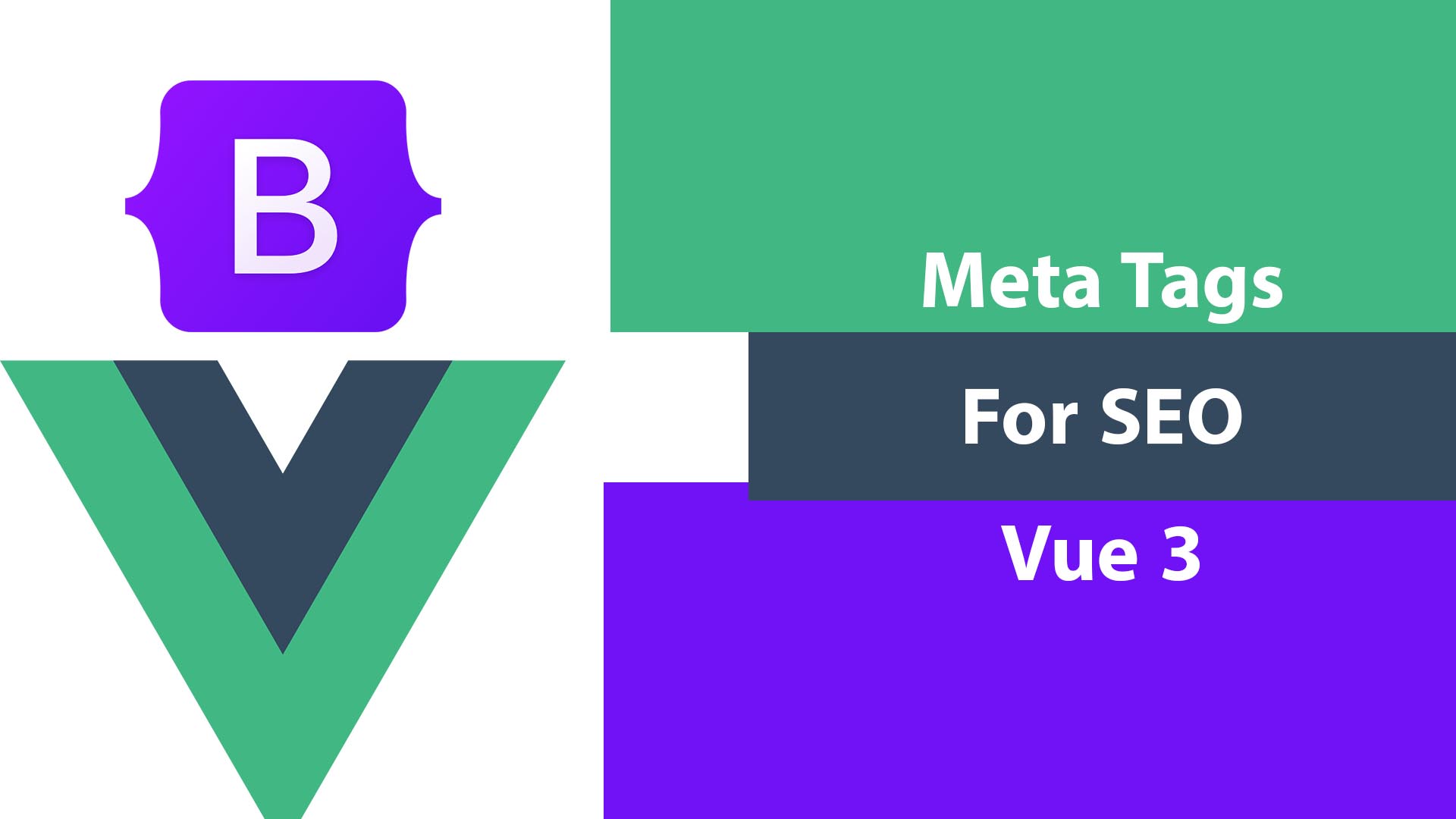
Let’s create a Head tag with custom Meta tags for SEO in SPA Vue.js 3, a single-page website. No server rendering is required.
Nowadays Google robots can crawl and index SPA website pages. Yes, the robot waits until the page loads. So, we should customize head meta tags for every page to give it a title, a description, and all the SEO meta tags. so let’s get started.
I have Vite Vue 3 project with bootstrap 5 that I use for this tutorial, I will leave tutorials links for this project below:
Here is the project structure I have two pages “Home” and “About”, we need to give them title tags and descriptions.
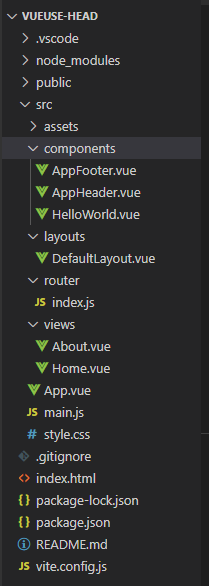
Installing vueuse/head
Let’s install “vueuse/head” package in our project, which will make it easy for creating and overriding head meta tags for each page.
npm i @vueuse/head
The second step is we need to add it to our Vue app in src\main.js
//
import { createHead } from '@vueuse/head'
const head = createHead()
createApp(App).use(head).use(router).mount('#app')
Adding Meta Tags
First, we create default meta tags for all pages then we can override them on any page we want. Let’s create head
in src\App.vue
<template>
<router-view/>
</template>
<script>
import {computed, reactive } from 'vue'
import { useHead } from '@vueuse/head'
export default ({
setup() {
const siteData = reactive({
title: `My website`,
description: `My beautiful website`,
})
useHead({
// Can be static or computed
title: computed(() => siteData.title),
meta: [
{
name: `description`,
content: computed(() => siteData.description),
},
],
})
},
})
</script>
Now, if you go to any page on the website you will find the title in the browser tab is changed to “My website” and if you inspect <head>
tag in the developer tool of the browser you should see that:
<meta name="description" content="My beautiful website">
Overriding Meta Tags In Vue 3
Let’s make the search engine happy and add title
and description
for the about page. We will add the following code in src\views\About.vue
<template>
<div class="container min-h-content py-5 text-center">
<div class="row py-lg-5">
<div class="col-lg-6 col-md-8 mx-auto">
<i class="bi bi-window-fullscreen h1"></i>
<h1>This is about </h1>
<router-link :to="{name:'Home'}"> to home page</router-link>
</div>
</div>
</div>
</template>
<script>
import { useHead } from '@vueuse/head'
export default ({
setup() {
useHead({
// Can be static or computed
title: 'About',
meta: [
{
name: `description`,
content: 'this about about page',
},
],
})
},
})
</script>
Here we just used useHead
for overriding the title and description, now you can test in the browser and see the difference.
You can add more meta tags for Facebook and Twitter as you want by the same way of adding the description. That’s all, I hope that was useful, thank you.