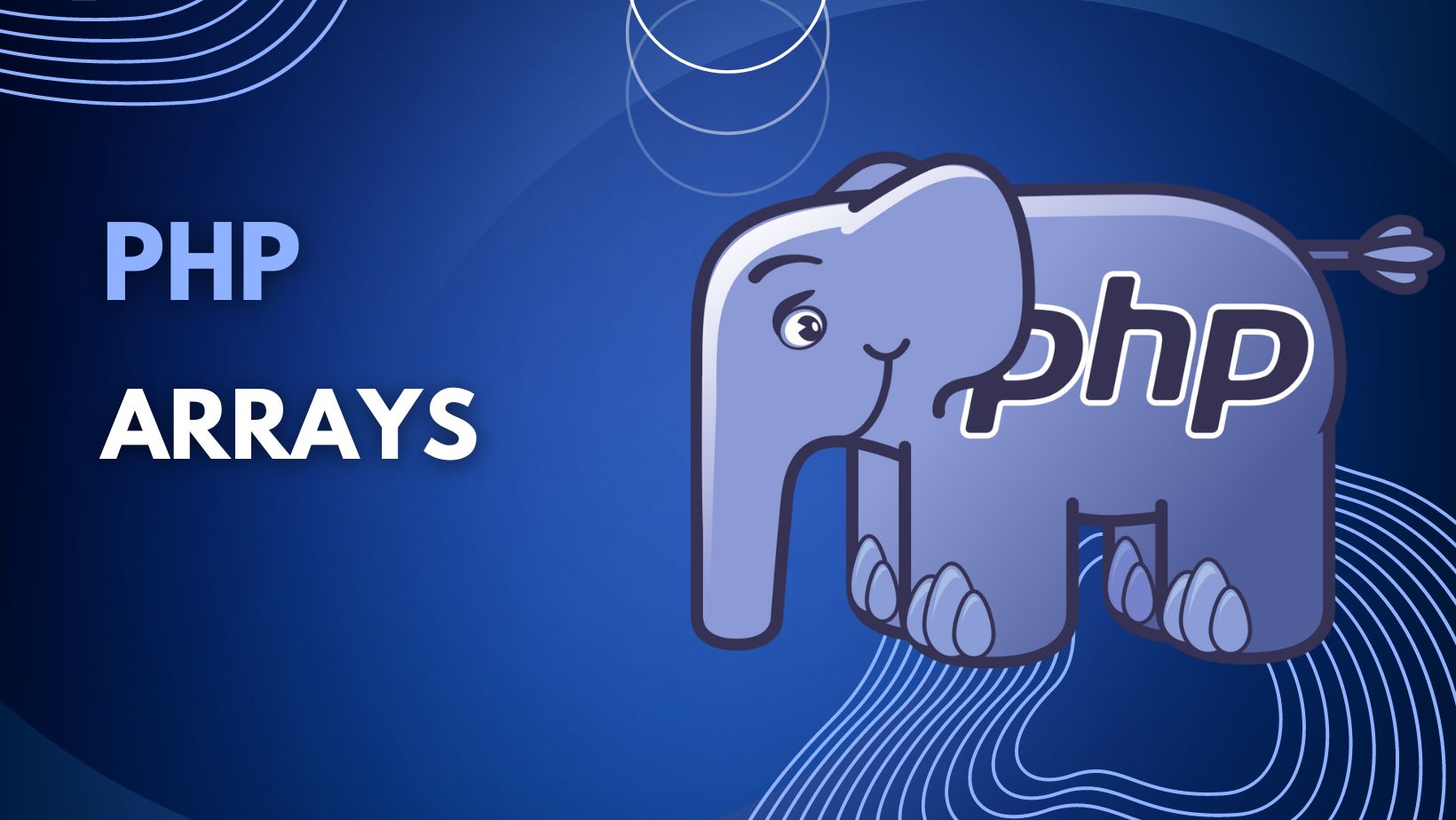
Learn PHP Arrays and its functions in this comprehensive guide. This tutorial covers everything you need to know about PHP Arrays, from creating and accessing arrays to sorting and searching arrays.
An array in PHP is a data structure that can store multiple values of the same or different data types in one single variable.
Cool Things To Do With The PHP String Functions | Learn PHP #02
Essential Functions To Deal With Numbers in PHP | Learn PHP #03
PHP Arrays
Arrays are created using the array()
function or you can create an array in PHP with the []
syntax. This is called the short array syntax.
$cars = array("Mercedes", "BMW", "Toyota");
//or
$cars = ["Mercedes", "BMW", "Toyota"];
We have created the same array of car brands in two different methods. This array holds string values for each index. What is an index? An index is a number that identifies the position of an element in an array.
The index of the first element Mercedes
is 0. The index of the second element BMW
is 1. The index of the third element Toyota
is 2. We can use the index to access the element of an array as the following code
$cars = ["Mercedes", "BMW", "Toyota"];
echo $cars[0]; //Mercedes
The type of this array is Indexed arrays because we use numeric indexes to access their elements. There are two more types of arrays Associative arrays and Multidimensional arrays.
Associative arrays use named keys to access their elements. The keys can be any type of string. Let’s create an array to show us the number of available cars for sale in each brand.
$cars = ["Mercedes" => 3, "BMW" => 4, "Toyota" => 5];
echo $cars['BMW']; //4
The Associative arrays store data in key-value pairs $array = [key => value,]
. The key is a unique identifier for the value, and the value can be of any data type.
Multidimensional arrays are arrays that contain other arrays, one or more arrays. The elements of a multidimensional array can be indexed using a combination of numeric and named keys.
$cars = [
"cool" => ["Mercedes", "BMW"],
"family" => ["Toyota", "Honda"]
];
echo $cars['cool'][1]; //BMW
The cool
key in the $cars
array points to an array with two elements: “Mercedes” and “BMW”. The 1
index in the cool array points to the second element, which is “BMW”.
Here is another example
$cars = [
["Volvo", "BMW", "Toyota"],
["Honda", "Mercedes", "Kia"]
];
echo $cars[1][2]; //Kia
The 1
index in the $cars
array points to the second array. The 2
index in the cars
array points to the third element in the second row, which is “Kia”.
The most used PHP array functions
is_array()
is_array() is used to check if a variable is an array. The function returns a boolean data type, true if the variable is an array, and false otherwise.
<?php
$cars = ["Mercedes", "BMW", "Toyota"];
if (is_array($cars)) {
echo "The variable cars is an array.";
} else {
echo "The variable cars is not an array.";
}
?>
count()
The count()
function in PHP is used to count the number of elements in an array
<?php
$cars = ["Mercedes", "BMW", "Toyota"];
echo count($cars); //3
?>
array_push()
This function adds an element to the end of an array.
<?php
$cars = ["Mercedes", "BMW", "Toyota"];
array_push($cars,"Kia");
echo $cars[3];//kia
?>
The array_push()
function adds the string “Kia” to the end of the cars
array. The echo $cars[3];
code will print the value of the element at the index 3
in the cars
array, which is “Kia”.
in_array()
in_array() checks if an element exists in an array.
<?php
$cars = ["Volvo", "BMW", "Toyota"];
if (in_array("Honda",$cars)) {
echo "Yes";
} else {
echo "No";
}
?>
More functions to modify arrays
- array_pop() : Removes the last element from an array and returns it.
- array_shift() : Removes the first element from an array and returns it.
- array_unshift() : Adds an element to the beginning of an array.
- array_slice() : Returns a slice of an array.
- array_merge() : Merges two or more arrays.
- array_reverse() : Reverses the order of an array.
Here example with an explanation of using these functions. We will use The print_r()
function to print the contents of a variable in a human-readable format. The function can be used to print the contents of any variable, including arrays, objects, and strings.
// Remove the last element from the array and print it
$cars = ["Volvo", "BMW", "Toyota"];
$last_car = array_pop($cars);
echo $last_car; // Toyota
// Remove the first element from the array and print it
$cars = ["Volvo", "BMW", "Toyota"];
$first_car = array_shift($cars);
echo $first_car; // Volvo
// Add the element "Honda" to the beginning of the array
$cars = ["Volvo", "BMW", "Toyota"];
array_unshift($cars, "Honda");
print_r($cars); // Array ( [0] => Honda [1] => Volvo [2] => BMW [3] => Toyota )
// Returns the slice of the array starting from the 2nd element to the 4th element
$cars = ["Volvo", "BMW", "Toyota", "Honda", "Mazda"];
$slice = array_slice($cars, 1, 3);
print_r($slice); // Array ( [1] => BMW [2] => Toyota )
// Merges the two arrays $cars and $trucks
$cars = ["Volvo", "BMW", "Toyota"];
$trucks = ["Ford", "Chevy", "Dodge"];
$merged_array = array_merge($cars, $trucks);
print_r($merged_array); // Array ( [0] => Volvo [1] => BMW [2] => Toyota [3] => Ford [4] => Chevy [5] => Dodge )
// Reverses the order of the array
$cars = ["Volvo", "BMW", "Toyota"];
array_reverse($cars);
print_r($cars); // Array ( [0] => Toyota [1] => BMW [2] => Volvo )
PHP sort functions for arrays
sort()
: Sorts an array in ascending order.rsort()
: Sorts an array in descending order.asort()
: Sorts an associative array in ascending order, according to the value.arsort()
: Sorts an associative array in descending order, according to the value.ksort()
: Sorts an associative array in ascending order, according to the key.krsort()
: Sorts an associative array in descending order, according to the key.
$numberArray = [8, 51, 20, 7, 3];
sort($numberArray);
print_r($numberArray); //Array ( [0] => 3 [1] => 7 [2] => 8 [3] => 20 [4] => 51 )
The other functions can be used as the example above.
useful information for you thank you.
Learn more: The Most Used PHP Operators | Learn PHP #05