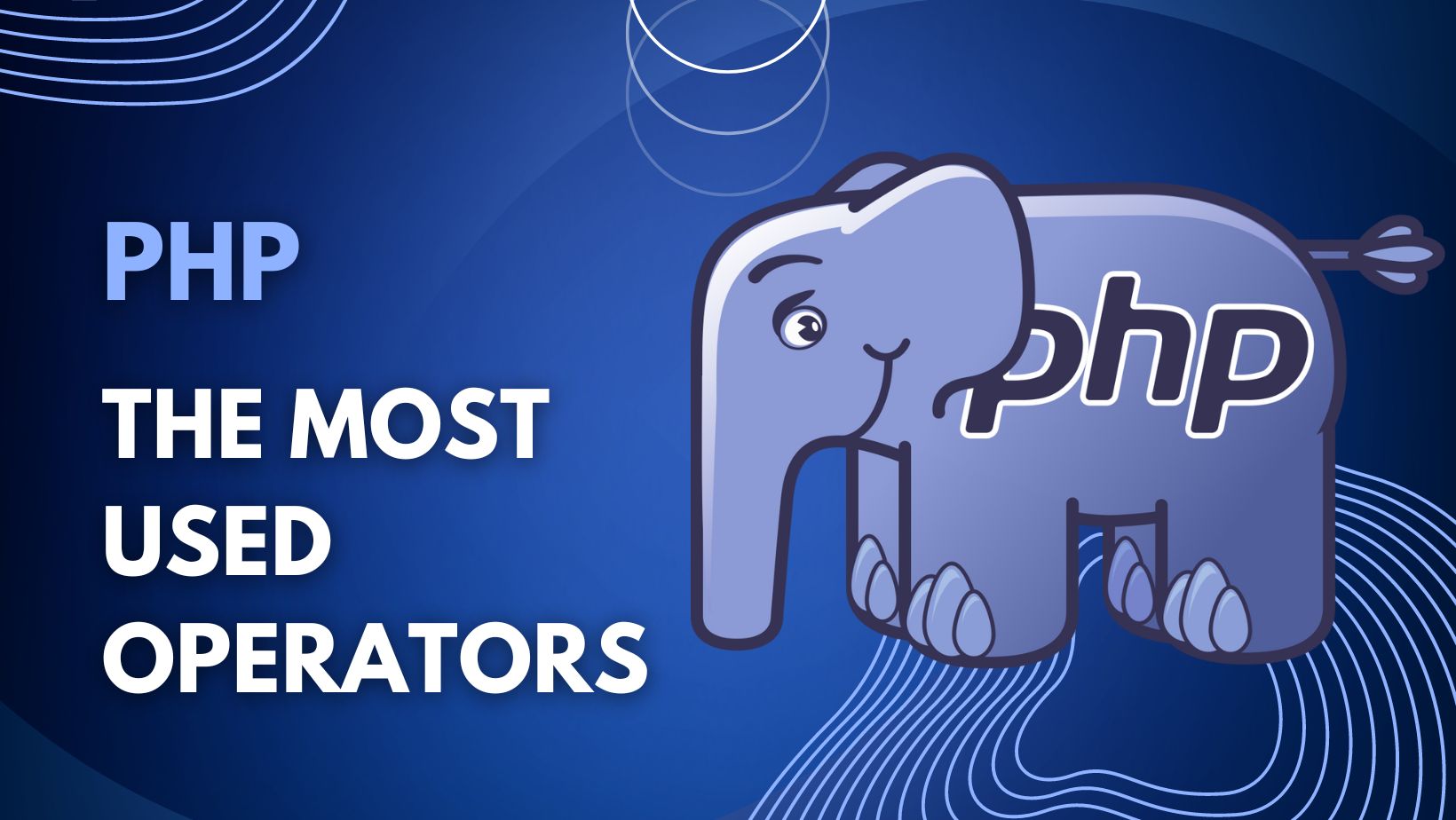
Learn The most used PHP operators. There are a lot of operators in PHP but what you need to know for a start is a few. we will explain the most used in real-life php code unless you make something uncommon.
Arithmetic operators
You must know the most common arithmetic operators as we use them a lot to perform mathematical operations on numbers. those operators are:
- Addition (+): Adds two or more operands.
- Subtraction (-): Subtracts the second operand from the first.
- Multiplication (*): Multiplies operands.
- Division (/): Divides the first operand by the second.
- Modulus (%): Divides the first operand by the second and returns the remainder.
- Exponentiation (**): Raises the first operand to the power of the second operand.
<?php
$x = 8;
$y = 2;
echo $x + $y ."<br>"; //10
echo $x - $y ."<br>"; //6
echo $x * $y ."<br>"; //16
echo $x / $y ."<br>"; //4
echo $x % $y ."<br>"; //0
echo $x ** $y ."<br>"; //64
?>
The code assigns the values 8 and 2 to the variables $x
and $y
, respectively. Then, it uses the arithmetic operators to perform the different operations.
PHP Assignment Operators
PHP has a set of assignment operators that can be used to assign the value of an expression to a variable
- =: Assigns the value of the expression to the variable.
- +=: Adds the value of the expression to the variable and assigns the result to the variable.
- -=: Subtracts the value of the expression from the variable and assigns the result to the variable.
- *=: Multiplies the value of the expression by the variable and assigns the result to the variable.
- /=: Divides the value of the expression by the variable and assigns the result to the variable.
- %=: Modulus the value of the expression by the variable and assigns the result to the variable.
<?php
$x = 5;
$x += 1; // $x = $x + 1 = 6
$x -= 1; // $x = $x - 1 = 5
$x *= 2; // $x = $x * 2 = 10
?>
PHP Increment & Decrement Operators
These cool operators are magic you will use them a lot, these operators are used to increment or decrement the value of a variable by 1.
<?php
$x = 1;
echo $x++; //1
echo $x ; //2
echo $x-- ; //2
echo $x ; //1
?>
We assigned the value 1 to the variable $x
.
- The first echo statement prints the value of $x before it is incremented by 1 to be 2.
- The second echo statement prints the value of $x after it is incremented.
- The third echo statement prints the value of $x before it is decremented by 1. So, the value 2 is printed.
- The fourth echo statement prints the value of $x after it is decremented.
- The names of these operators are $x++ Postfix-increment and $x– Postfix-decrement.
- Here is another example but with Prefix-increment ++$x Prefix-decrement –$x
<?php
$x = 1;
echo ++$x; //2
echo $x; //2
echo --$x; //1
echo $x ; //1
?>
The code assigns the value 1 to the variable $x
. Then, it uses the prefix increment and decrement operators to print the value of $x
.
The prefix increment or decrement operator increments or decrements the value of the variable first, and then returns the value of the variable.
PHP Comparison and Logical Operators
PHP has a set of logical and Comparison operators that can be used to control the flow of execution of a program.
PHP comparison operators are used to compare two values and return a Boolean value (true or false).
- Equal to (==): Returns true if the two values are equal.
- Not equal to (!=): Returns true if the two values are not equal.
- Greater than (>): Returns true if the first value is greater than the second value.
- Greater than or equal to (>=): Returns true if the first value is greater than or equal to the second value.
- Less than (<): Returns true if the first value is less than the second value.
- Less than or equal to (<=): Returns true if the first value is less than or equal to the second value.
We usually use them with conditional statements such as if
and else
to allow us to execute different code blocks depending on the value of a condition.
if (condition) {
code block to be executed if the condition is true;
} else if (condition) {
code block to be executed if the condition is true;
} else {
code block to be executed if all of the previous IF and ELSEIF statements are false;
}
let’s make an example
<?php
$x = 5;
$y = 6;
if ($x > $y) {
echo "The value of x is greater than 6";
} else {
echo "The value of x is not greater than 6";
}
?>
<?php
$animal = 'dog';
if ($animal == 'cat') {
echo "meow, meow,";
} else if ($animal == 'dog'){
echo "woof, woof,";
} else {
echo "It's not a cat or a dog";
}
?>
What if you want to combine multiple conditions?! like if the animal is a cat or dog print “a pet” else print “we don’t know”.
<?php
$animal = 'dog';
if ($animal == 'cat' or $animal == 'dog') {
echo "a pet";
} else {
echo "we don't know";
}
?>
IF
statement checks if the value of the variable $animal
is equal to “cat” or “dog”.
PHP has a set of logical operators that can be used to combine two or more logical expressions. The most common logical operators in PHP are:
- AND (&&): Returns true if both expressions are true.
- OR (||): Returns true if either expression is true.
- XOR (xor): Returns true if either expression is true, but not both.
- NOT (!): Reverses the logical value of an expression.
You can write the operator in multiple formats such as in uppercase AND
or lowercase and
or &&
<?php
$x = 5;
if ($x != 4 && $x != 8) { //and
echo "true";
}
if ($x == 5 || $x == 8) { //or
echo "true";
}
if ($x == 5 || $x == 8 || $x == 10) { //or
echo "true";
}
?>
Ternary operator
The ?:
operator is a ternary operator in PHP, The ternary operator is a shorthand for the if else
statement but it takes only one condition at a time which means it takes three operands. The first operand is the condition, the second operand is the value if the condition is true, and the third operand is the value if the condition is false.
condition ? value_if_true : value_if_false
Here is an example:
<?php
$age = 18;
$message = $age >= 18 ? "You are an adult." : "You are a minor.";
echo $message;
?>
The value of $message
will be “You are an adult.” If the variable $age >= 18
. Otherwise, the value of $message
will be “You are a minor.”.
Here is the same example but with IF ELSE
<?php
$age = 18;
if( $age >= 18){
$message = "You are an adult." ;
}else{
$message = "You are a minor.";
}
echo $message;
?>
The ?: operator is a concise way to write conditional statements. It can be used to replace if-else statements in many cases. It is a powerful tool that can be used in many different ways and can help you write concise and efficient code.
Here is another example with a boolean variable.
<?php
$is_logged_in = true;
$message = $is_logged_in ? "You are logged in." : "Please login.";
echo $message;
?>
The value of $message
will be “You are logged in.” if the variable $is_logged_in
is true. Otherwise, the value of $message
will be “Please login.”.
Learn more: Everything you need to know About PHP Ternary operator ?:
Conclusion
PHP operators are symbols that are used to perform operations on variables and values. They are divided into different categories, such as arithmetic operators, assignment operators, comparison operators, logical operators, string operators, and array operators. Learn more in the PHP Official Manual