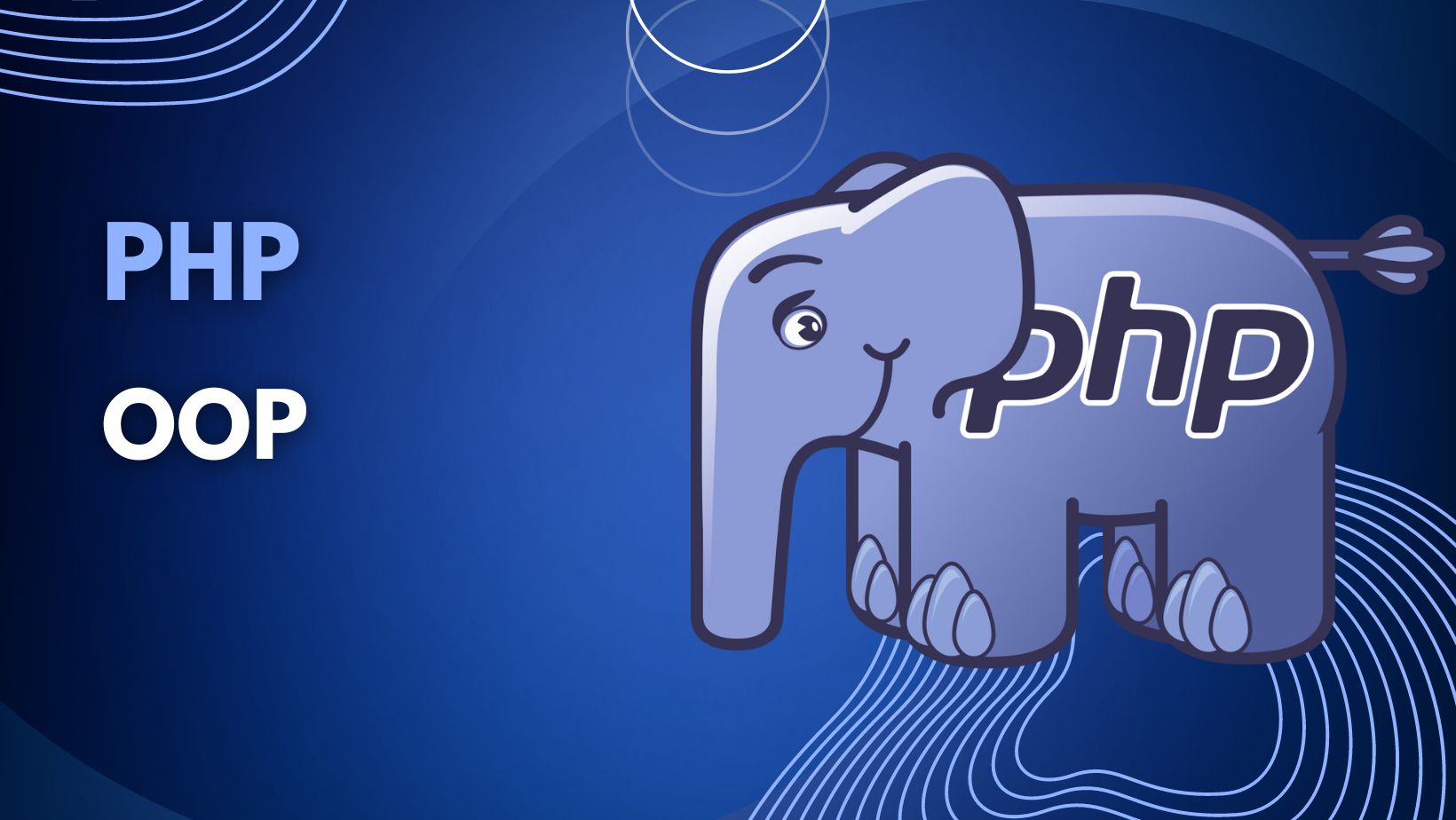
Learn how to create object-oriented PHP applications with this comprehensive tutorial. OOP is a powerful programming paradigm that can be used to create complex and sophisticated software.
Everything in this world is an object, people, cars, TVs, etc. These objects have properties and methods for their behaviors and every object has a blueprint that makes you identify what it is. When we bring that concept to our code and make every piece of code an object, this is OOP, Object-Oriented Programming.
PHP OOP Example
Let’s make an application about selling cars. Can you tell the objects in this app?
You are right, we have a car object, a customer object, a seller object, etc. Let’s code our car object but first, we need to understand a few things, the blueprint as we said everything has a blueprint that makes you identify what it is. so here the blueprint means class, We will create a car class then we make an object.
- Classes: A class is a blueprint for creating objects. It defines the properties and methods of an object.
- Objects: An object is an instance of a class. It has the properties and methods defined in the class.
That means if we make an instance of the car class we will have an object. BMW, and Mercedes cars are objects from the car class, the same thing goes for the customer class the object will be John Doe, Ahmed.
Let’s demonstrate that in codes
<?php
//creating car class
class Car {
public $brand;
public $color;
public $type;
public $price;
public function __construct($brand, $color, $type, $price) {
$this->brand= $brand;
$this->color= $color;
$this->type= $type;
$this->price= $price;
}
public function getInfo() {
return "<br> Brand: {$this->brand},
<br> Color: {$this->color},
<br> Type: {$this->type},
<br> price: {$this->price}$";
}
}
// making an object
$bmw = new Car('BMW','red','Sedan','56000');
echo $bmw->getInfo();
?>
Let’s explain the code
We define a PHP class called Car
class Car {
//code here
}
This class has four properties: brand, color, type, and price. A property is a variable that belongs to an object.
class Car {
public $brand;
public $color;
public $type;
public $price;
//...
}
We want to make these four properties have values when we create an instance of the class. So we need to create a construct function. The __construct() method is a special method in PHP that is called when an object is created. It is used to initialize the object’s properties.
class Car {
public $brand;
public $color;
public $type;
public $price;
public function __construct($brand, $color, $type, $price) {
$this->brand= $brand;
$this->color= $color;
$this->type= $type;
$this->price= $price;
}
}
__construct
method takes four parameters: the brand, color, type, and price of the car. The code first assigns the value of the $brand
parameter to the $this->brand
property.
Note when we use the class properties inside the class we change them to $this->VARIABLE_NAME_WITHOUT_$
as we did with $brand
.
The value of the $color
parameter to the $this->color
property. The $this->color
property is also a public property of the Car
class which means we can use it outside of the class. The same happens with $type
and $price
.
The public
keyword is used to declare the properties and methods of the class. This means that they can be accessed from outside the class.
The this
keyword is used to refer to the current object.
The equal sign (=) is used to assign a value to a property.
class Car {
//..
public function getInfo() {
return "<br> Brand: {$this->brand},
<br> Color: {$this->color},
<br> Type: {$this->type},
<br> price: {$this->price}$";
}
}
getInfo()
function returns a string with information about the car, including the brand, color, type, and price.
We created an object as the following.
$bmw = new Car('BMW','red','Sedan','56000');
echo $bmw->getInfo();
/* output:
Brand: BMW,
Color: red,
Type: Sedan,
price: 56000$
*/
The code created a new object of the Car class and assigned it the values “BMW”, “red”, “Sedan”, and “56000”. It will print the information of the object using the getInfo()
method.
Now we can create more objects for other cars.
$bmw = new Car('BMW','red','Sedan','56000');
echo $bmw->getInfo();
$fiat = new Car('fiat','black','Hatchback','2600');
echo $fiat->getInfo();
$honda = new Car('Honda','white','SUV','12600');
echo $honda->color;
Access Modifiers
Access modifiers are keywords in PHP that control the accessibility of properties and functions in a class. There are three access modifiers in PHP:
public: The public access modifier makes a property or method accessible from anywhere. This is the default access modifier.
protected: The protected access modifier makes a property or method accessible from within the class and from classes that inherit from the class.
private: The private access modifier makes a property or method accessible only from within the class.
PHP OOP Inheritance
Inheritance is a feature of object-oriented programming (OOP) that allows a new class to inherit the properties and methods of an existing class. This means that the child class can reuse the code of the parent class, which can help to reduce code duplication.
Let’s inherit our car class to a new class about sports cars.
<?php
class Car {
public $brand;
public $color;
public $type;
public $price;
public function __construct($brand, $color, $type, $price) {
$this->brand= $brand;
$this->color= $color;
$this->type= $type;
$this->price= $price;
}
public function getInfo() {
return "<br> Brand: {$this->brand},
<br> Color: {$this->color},
<br> Type: {$this->type},
<br> price: {$this->price}$";
}
}
Class SportsCar extends Car {
public $horsepower;
Public $from0To100;
public function __construct($brand, $color, $price, $horsepower, $from0To100) {
parent::__construct($brand, $color, 'Sports car', $price);
$this->horsepower = $horsepower;
$this->from0To100 = $from0To100;
}
public function getInfo(){
$info = parent::getInfo();
return "{$info} <br> HP: {$this->horsepower}, <br> 0 To 100KM: {$this->from0To100},";
}
}
$ferrari = new SportsCar('Ferrari','red','560000',780, 3.5);
echo $ferrari->getInfo();
/* output:
Brand: Ferrari,
Color: red,
Type: Sports car,
price: 560000$
HP: 780,
0 To 100KM: 3.5,
*/
echo "<br> {$ferrari->color}";
?>
The SportsCar
class inherits from the Car
class and adds two more properties: horsepower and from0To100.
The __construct()
method of the SportsCar
class calls the __construct()
method of the Car
class parent::__construct($brand, $color, 'Sports car', $price);
to initialize the properties of the Car
class. It then initializes the $horsepower
and $from0To100
properties of the SportsCar
class.
The getInfo()
method of the SportsCar
class overrides and gets the information from the getInfo()
method of the Car
class parent::getInfo();
. It then adds the horsepower and from0To100 information to the string.
We created an object of the SportsCar class named $ferrari and assigned it the values “Ferrari”, “red”, “560000”, 780, and 3.5. You then printed the information of the object using the getInfo() method.
Let’s add another function to SportsCar
class, engine_type()
that takes an integer as a parameter. The method checks the value of the parameter and then increases the price of the car by 1000 if the parameter is 1, or by 2000 if the parameter is 2 or not 1. The method then returns the price of the car.
Class SportsCar extends Car {
// previous code...
public function engine_type($number){
$number == 1? $this->price += 1000 : $this->price += 2000;
return $this->price;
}
}
$ferrari = new SportsCar('Ferrari','red','560000',780, 3.5);
echo $ferrari->engine_type(1);
The final Keyword
The final keyword in PHP is used to prevent a class, method, or property from being overridden by a child class.
- final class: A final class cannot be extended by child classes.
- final method: A final method cannot be overridden by a child class.
- final property: A final property cannot be changed by a child class.
Let’s add the final keyword to our Car class and try to inherit it.
final class Car {
//code ...
}
Class SportsCar extends Car {
//code ...
}
We will get an error;
Fatal error: Class SportsCar cannot extend final class Car in index.php on line 24
PHP Static Methods & Static Properties
Static methods and properties are special methods and properties that are associated with a class, not with an object of the class. This means that they can be accessed without creating an object of the class.
Static methods are declared with the static
keyword. Static properties are declared with the static
keyword.
class Car {
public static $brand = "Honda";
public static function getHorsePower() {
return 170;
}
public static function getBrand() {
return self::$brand;
}
}
echo Car::$brand;
echo Car::getHorsePower();
echo Car::getBrand();
The static property $brand
can also be accessed from within a static method. The self
keyword refers to the current class. In this case, the self
keyword refers to the Car
class.
PHP Interfaces
An interface defines the methods that a class must implement. A class that implements an interface must provide an implementation for all of the methods in the interface. they cannot have properties and all of their methods must be public.
Interfaces are used to:
- Define the behavior of a class without specifying how that behavior is implemented.
- Allow for polymorphism, which is the ability to treat different objects of different types in the same way.
- Promote code reuse by allowing different classes to implement the same interface.
interface CarInterface {
public function getBrand();
public function getHorsePower();
}
class Car implements CarInterface {
public function getBrand() {
echo "Honda";
}
public function getHorsePower() {
echo 174;
}
}
$honda= new Car ();
$honda->getBrand();
The CarInterface
interface defines two methods: getBrand()
and getHorsePower()
. Any class that implements the CarInterface
must provide an implementation for both of these methods.
keep in mind about interfaces in PHP:
- Interfaces cannot have constructors or destructors.
- Interfaces cannot be instantiated.
- Interfaces can extend other interfaces.
- Classes can implement multiple interfaces.
Abstract Classes
An abstract class defines the methods that a class can implement. A class that extends an abstract class can choose to implement some or all of the methods in the abstract class. An abstract class is a class that contains at least one abstract method. An abstract method is a method that is declared, doesn’t have code and it must be coded in the child class. An abstract class or method is defined by the abstract
keyword.
abstract class CarAbstract {
public abstract function getBrand();
public abstract function getHorsePower();
}
class Car extends CarAbstract {
public function getBrand() {
echo "Honda";
}
public function getHorsePower() {
echo 174;
}
}
$carModel1= new Car ();
$carModel1->getBrand();
The CarAbstract
class defines two methods: getBrand()
and getHorsePower()
. However, the CarAbstract
class does not provide an implementation for these methods. Instead, it leaves it up to the child classes to provide an implementation.
It is important to use abstract classes wisely in PHP, they can make code more complex.
Learn more about PHP Interfaces and Abstract Classes
PHP Destructor
In PHP, a destructor is a function that is called when an object is destroyed. The destructor is called automatically by PHP, and it is a good place to do cleanup tasks, such as releasing resources or closing files.
The destructor function is defined using the __destruct() function. The __destruct() function is a magic function, which means that it is automatically called by PHP when the object is destroyed.
class car {
public $brand;
public function __construct($brand) {
$this->brand = $brand;
}
public function __destruct() {
echo "Destructor called for this car {$this->brand}.";
}
}
$bmw = new car("BMW");
echo $bmw->brand;
unset($bmw); // This will call the destructor function.
?>
The __destruct()
function in the Car
class is called when the bmw
object is destroyed. The destructor function prints a message to the console that says “Destructor called for this car BMW.”
The unset()
function is used to destroy an object. When an object is destroyed, its memory is released.
In your code, the unset()
function is called after the echo
statement. This means that the echo
statement will still be executed, even though the bmw
object has been destroyed.
Conclusion
OOP is a powerful programming paradigm that can be used to create complex and sophisticated applications. It is a must-know for any serious PHP developer.