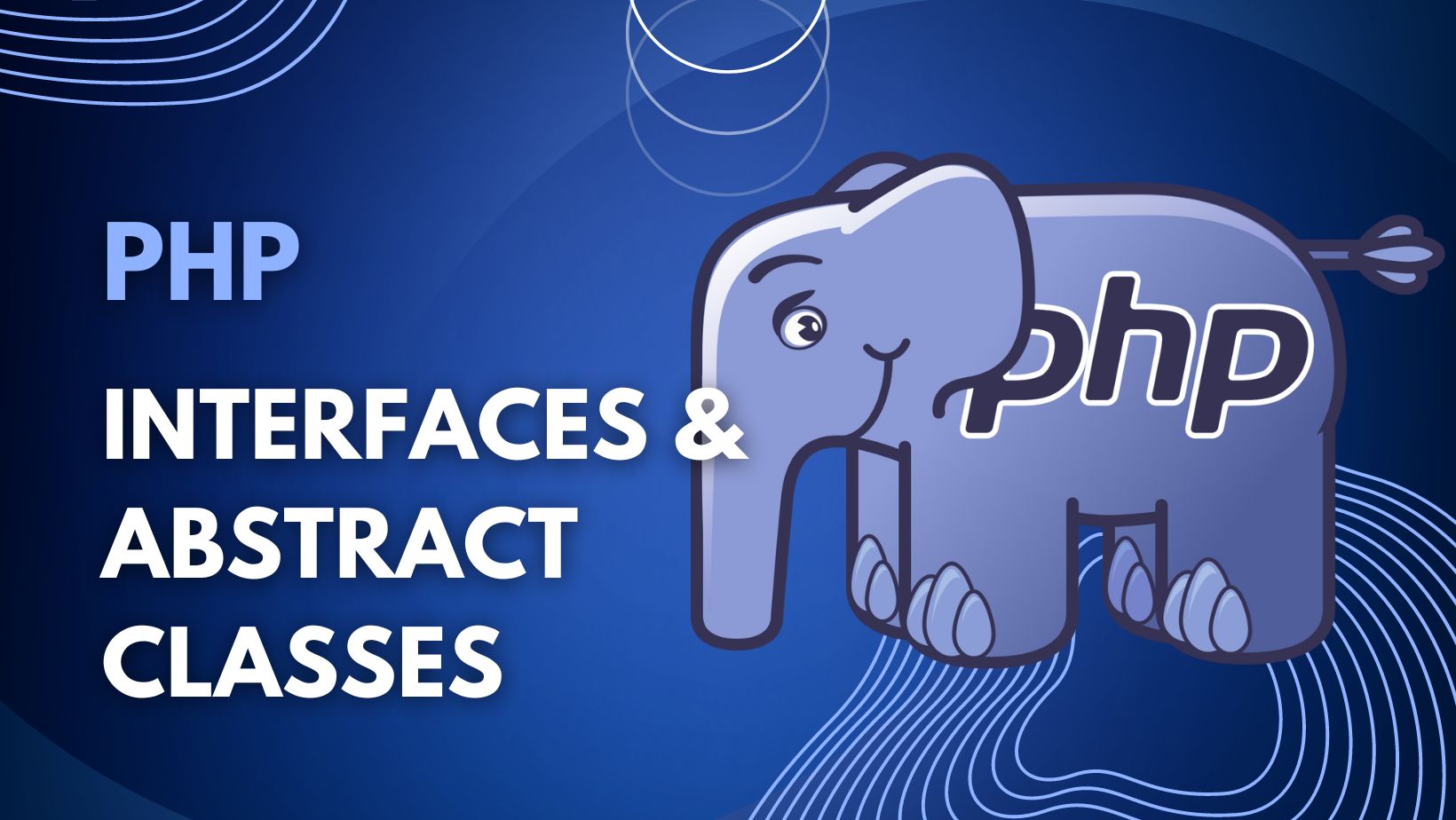
In PHP, interfaces and abstract classes are both used to define the structure of a class. However, they have different purposes.
Learn more about PHP Object-Oriented Programming OOP
Abstract Classes
An abstract class defines the methods that a class can implement. A class that extends an abstract class can choose to implement some or all of the methods in the abstract class. An abstract class is a class that contains at least one abstract method. An abstract method is a method that is declared, doesn’t have code and it must be coded in the child class. An abstract class or method is defined with the abstract
keyword.
abstract class CarAbstract {
public abstract function getBrand();
public abstract function getHorsePower();
}
class Car extends CarAbstract {
public function getBrand() {
echo "Honda";
}
public function getHorsePower() {
echo 174;
}
}
$carModel1= new Car ();
$carModel1->getBrand();
The CarAbstract
class defines two methods: getBrand()
and getHorsePower()
. However, the CarAbstract
class does not provide an implementation for these methods. Instead, it leaves it up to the child classes to provide an implementation.
It is important to use abstract classes wisely in PHP, they can make code more complex.
Here are some of the benefits of using abstract classes in PHP:
- They can be used to define common functionality that can be inherited by other classes.
- They can help to reduce code duplication.
- They can make code more modular and easier to maintain.
Abstract class example
abstract class CarAbstract {
public $brand;
public function __construct($brand) {
$this->brand = $brand;
}
public function getSpeed(){
echo 350;
}
public abstract function getBrand();
public abstract function getHorsePower();
}
class car extends CarAbstract {
public function getBrand() {
echo $this->brand;
}
public function getHorsePower() {
echo 174;
}
}
$carModel1= new car ('BMW');
$carModel1->getBrand();
$carModel1->getSpeed();
The CarAbstract
class is an abstract class because it contains two abstract methods, getBrand()
and getHorsePower()
. These methods must be implemented in any class that inherits from the CarAbstract
class.
The car
class inherits from the CarAbstract
class and implements the getBrand()
and getHorsePower()
methods. This means that the car
class can be instantiated and can be used to create car objects.
The getBrand()
method in the car
class simply returns the value of the $brand
property. The getHorsePower()
method in the car
class returns the value 174.
Interfaces
An interface defines the methods that a class must implement. A class that implements an interface must provide an implementation for all of the methods in the interface. they cannot have properties and all of their methods must be public.
Interfaces are used to:
- Define the behavior of a class without specifying how that behavior is implemented.
- Allow for polymorphism, which is the ability to treat different objects of different types in the same way.
- Promote code reuse by allowing different classes to implement the same interface.
interface CarInterface {
public function getBrand();
public function getHorsePower();
}
class Car implements CarInterface {
public function getBrand() {
echo "Honda";
}
public function getHorsePower() {
echo 174;
}
}
$honda= new Car ();
$honda->getBrand();
The CarInterface
interface defines two methods: getBrand()
and getHorsePower()
. Any class that implements the CarInterface
must provide an implementation for both of these methods.
keep in mind about interfaces in PHP:
- Interfaces cannot have constructors or destructors.
- Interfaces cannot be instantiated.
- Interfaces can extend other interfaces.
- Classes can implement multiple interfaces.
Interfaces vs Abstract Classes
- Abstract classes are used to define a common set of features and methods that can be inherited by concrete subclasses. This can help to reduce code duplication and make code more modular.
- Interfaces are used to define a contract that a class must agree to fulfill. This can help to ensure that different classes that are working together have a consistent set of features and methods.
which should you use, an interface or an abstract class? The answer depends on the specific requirements of your situation. If you need to define the behavior of a class without specifying its implementation, then you should use an interface. If you need to define the behavior of a class and its implementation, then you should use an abstract class.