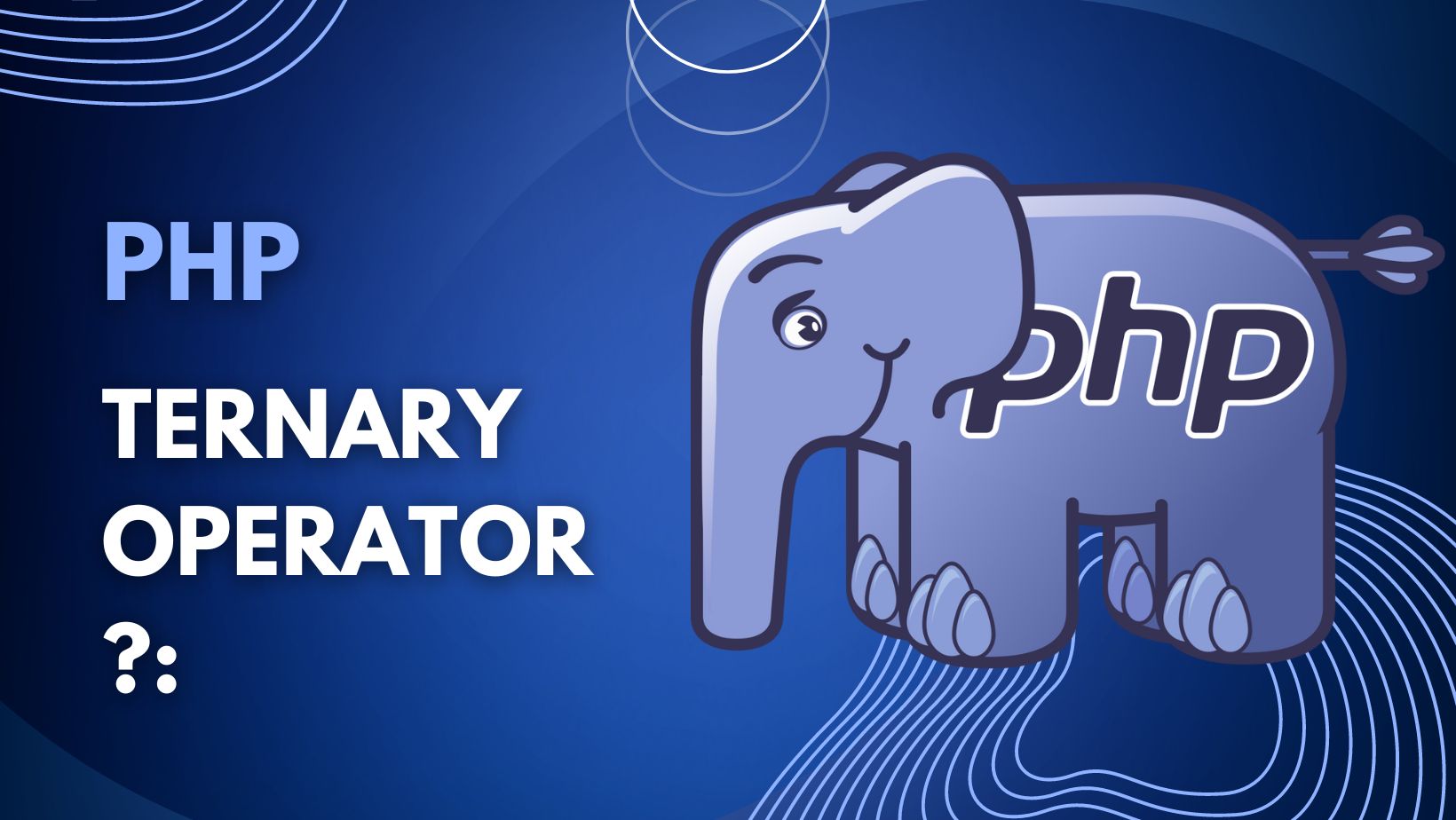
The ?:
operator is a ternary operator in PHP, The PHP ternary operator is a conditional operator that can be used to replace an if-else statement with a single line of code. It is shorter and more concise, but it is not as flexible and can be more difficult to read. This article explains everything you need to know about PHP ternary operators, including their syntax, how to use them, and when to use them.
PHP Ternary operator syntax
The ?:
operator is a ternary operator in PHP, The ternary operator is a shorthand for the if else
statement but it takes only one condition at a time which means it takes three operands. The first operand is the condition, the second operand is the value if the condition is true, and the third operand is the value if the condition is false.
condition ? value_if_true : value_if_false
PHP Ternary operator examples
<?php
$age = 18;
$message = $age >= 18 ? "You are an adult." : "You are a minor.";
echo $message;
?>
The value of $message
will be “You are an adult.” If the variable $age >= 18
. Otherwise, the value of $message
will be “You are a minor.”.
Here is the same example but with IF ELSE
<?php
$age = 18;
if( $age >= 18){
$message = "You are an adult." ;
}else{
$message = "You are a minor.";
}
echo $message;
?>
The ?: operator is a concise way to write conditional statements. It can be used to replace if-else statements in many cases. It is a powerful tool that can be used in many different ways and can help you write concise and efficient code.
Here is another example with a boolean variable.
<?php
$is_logged_in = true;
$message = $is_logged_in ? "You are logged in." : "Please login.";
echo $message;
?>
The value of $message
will be “You are logged in.” if the variable $is_logged_in
is true. Otherwise, the value of $message
will be “Please login.”.
The shorthand ternary operator
The shorthand ternary operator is a simplified version of the ternary operator.
<?php
$name = 'John';
$username = $name ?: 'guest';
echo "Welcome, {$username}!";
?>
The condition is whether the variable $name
is empty. If it is empty, the value of $username
will be guest
. Otherwise, the value of $username
will be the value of $name
.
In this case, the variable $name
is not empty, so the value of $username
will be $name
, which is “John”. The output of the code will be “Welcome, John!”.
It’s the same as this equivalent if-else statement
if (!empty($name)) {
$username = $name;
} else {
$username = 'guest';
}
echo "Welcome, {$username}!";
Chaining ternary operators
It is possible to chain ternary operators in PHP. Chaining ternary operators means using multiple ternary operators in a row to evaluate multiple conditions.
The syntax for chaining ternary operators is:
condition1 ? expression1 : (condition2 ? expression2 : expression3);
Let’s try that on the example of the age.
<?php
$age = 15;
$message = $age >= 18 ? "You are an adult." : ($age >= 16 ? "You are a teenager." : "You are a minor.");
echo $message
?>
The code first checks if the value of the variable $age
is greater than or equal to 18. If it is, then the message “You are an adult.” is printed. Otherwise, the code checks if the value of $age
is greater than or equal to 16. If it is, then the message “You are a teenager.” is printed. Otherwise, the message “You are a minor.” is printed.
In this case, the value of $age
is 15, so the message “You are a minor.” is printed.
Here is another example:
<?php
$is_logged_in = true;
$is_admin = false;
$message = $is_logged_in ? ($is_admin ? "You are an admin." : "You are a logged in user.") : "You are not logged in.";
echo $message
?>
The code first checks if the value of the variable $is_logged_in
is true. If it is, then the code checks if the value of the variable $is_admin
is also true. If it is, then the message “You are an admin.” is printed. Otherwise, the message “You are a logged in user.” is printed. Otherwise, the message “You are not logged in.” is printed.
Ternary operators vs. if-else statement
Ternary operators and if-else statements are both conditional statements that can be used to make decisions in code. However, there are some differences between them.
- Ternary operators are shorter and more concise. A ternary operator can be used to replace an if-else statement with a single line of code.
- Ternary operators are not as flexible. Ternary operators can only be used to make decisions with two outcomes. If you need to make a decision with more than two outcomes, you will need to use an if-else statement.
- Ternary operators can be more difficult to read. Ternary operators can be difficult to read if they are not well-formatted. It is important to make sure that the condition and the two outcomes are clearly separated.
In general, ternary operators should be used when you need to make a simple decision with two outcomes and you want to write concise code. If-else statements should be used when you need to make a more complex decision or when you need to make a decision with more than two outcomes.
Tips for using ternary operators
Here are some tips for using ternary operators effectively:
- Use them only when the decision is simple and the code is easy to read.
- Make sure that the condition and the two outcomes are clearly separated.
- Avoid using chaining ternary operators, it makes the code unreadable and hard to understand.
- Use comments to explain what the ternary operator is doing.
Here is an example:
// Print a message based on the user's age
$age = 15;
$message = $age >= 18 ? "You are an adult." : "You are a minor.";
Conclusion
Ternary operators are a conditional operator that can be used to replace an if-else statement with a single line of code. Ternary operators should be used when you need to make a simple decision with two outcomes and you want to write concise code. If-else statements should be used when you need to make a more complex decision or when you need to make a decision with more than two outcomes.
Learn more about The Most Used PHP Operators | Learn PHP #05