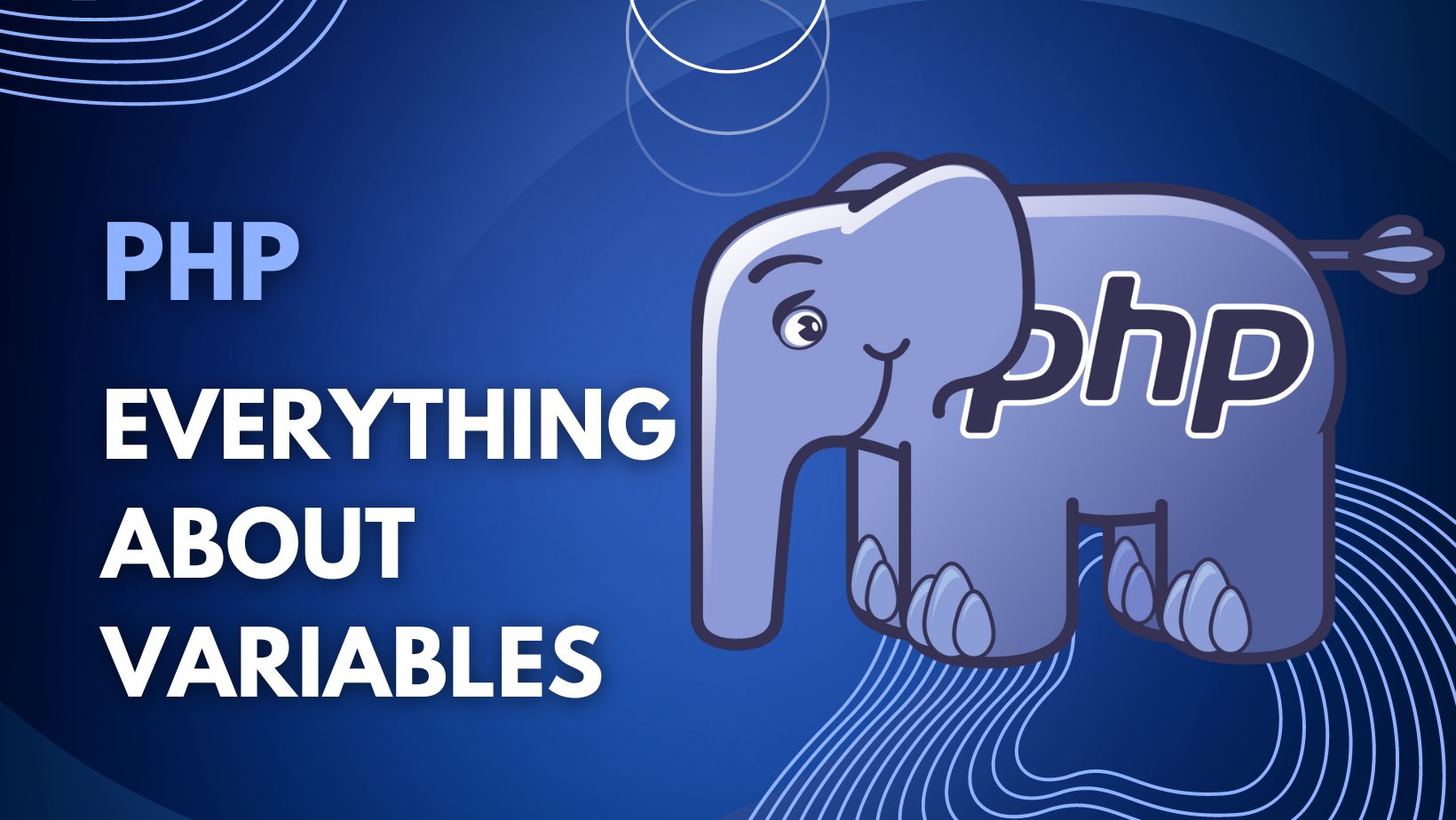
Learn PHP variables, declaring, deleting, scope, In addition to defining Constants and Global Variables and getting predefined variables Superglobals.
This tutorial is for beginners and for PHP developers who want to refresh their memory. if you want to know more about PHP in web development world check this article:
Is PHP Dead? Why PHP? Is PHP a popular language?
PHP variables
PHP variable is like a Microsoft Word file you write everything you want once and used it everywhere you want, you can copy part of the content or all the content, and you can add or remove its content. the Word file can contain text, numbers, images, articles, data, etc. In other words, the variable is the container to save data in it and it stores multiple data types that will talk about later.
Technically, the variable is a named location in memory that stores a value, Variables are created when they are first assigned a value, and they are destroyed when they are no longer needed.
In PHP, variables are declared using the $
sign, followed by the name of the variable,
<?php
$message = "Hello world!";
?>
Variable names are case-sensitive so $message
is different from $Message
. The name must start with a letter or the underscore character and it can only contain alpha-numeric characters and underscores.
<?php
$_age = 28;
$name = "John Doe";
$message2 = "Hello world!";
?>
This is how we declare variables in PHP. but what if you want to delete a variable? does PHP do that? this is an interview question.
The unset()
function is used to delete a variable and make the variable unavailable. It does not delete the data that is stored in the variable for example if you assigned a file path to the variable and deleted it with the unset()
function the file will not be deleted but the variable cannot be used anymore.
<?php
$message = "Hello world!";
echo $message;
unset($message);
echo $message;
?>
The echo
keyword to print the value of $message
“Hello, world!” to the screen. it will print it the first time but in the second echo it will not print because we deleted the variable with unset unset($message);
PHP Variables Scope
PHP has three different variable scopes to determine where the variable can be accessed.
Global variables
Global variables are created outside of any function or loop, and they are accessible from anywhere in the script but to access the global variable inside a function or loop, you must use the global
keyword before the variable name.
<?php
$message = "Hello world!";
function printMessage (){
global $message;
echo $message;
}
printMessage();
?>
Local variables
Unlike global variables, local variables are declared within a function or a loop, and they are only accessible within the function or loop which means you can’t use them outside.
<?php
$message = "Hello world!";
function printMessage (){
$message = "function message";
echo $message;
}
printMessage(); //it will print the local variable $message
echo '<br>'. $message; //it will print the global variable $message
?>
'<br>'.
this HTML tag to print $message
in a new line.
Static variables
A static variable in PHP is a variable that retains its value between function calls. This means that the value of a static variable is not lost when the function that it is declared in exits. to declare a static variable we use static
keyword before the variable name.
<?php
function myFunction() {
static $counter = 0;
$counter++;
echo $counter .'<br>';
}
myFunction(); // output is 1
myFunction(); // output is 2
?>
In this function we increment $counter
by 1 using $counter++;
then we print the $counter, The first time the myFunction() function is executed, the value of $counter is initialized to 0, and that is what usually happens without a static variable, but because we declared $counter
as static. The second time the myFunction()
function is executed, the value of $counter
is still 1, because it retained its value from the previous function call. The function then increments the value of $counter
and echo
it.
Here are some of the advantages of using static variables:
They can be used to store data that needs to be preserved between function calls and share data between functions.
Here are some of the disadvantages of using static variables:
They can make code more difficult to read and understand and less reusable and can lead to memory leaks.
Constants
A constant in PHP is a named identifier that represents a value that cannot be changed. Constants are declared using the const
keyword. Constants are case-sensitive and don’t use $
sign with the name. Constants are automatically global and can be used across the entire script.
<?php
const counter = 0;
function myFunction() {
echo counter;
}
myFunction();
?>
There is another method to declare a constant using define(name, value)
<?php
define("MESSAGE", "Hello, world!");
function myFunction() {
echo MESSAGE ;
}
myFunction(); // output: Hello, world!
?>
But you should use the first one const message
because it works both inside and outside of a class definition. we will explain more about classes in the OOP tutorial.
PHP Superglobal
Superglobal variables in PHP are predefined variables that are always accessible, regardless of the scope. You can access superglobal variables from anywhere in your PHP code.
The PHP superglobal variables are:
$GLOBALS
: A variable that contains all of the global variables in the script.$_SERVER
: A variable that contains information about the server environment.$_GET
: A variable that contains data that is passed to the script through the URL.$_POST
: A variable that contains data that is passed to the script through an HTML form.$_FILES
: A variable that contains information about files that are uploaded to the server through an HTML form.$_COOKIE
: A variable that contains cookies that are set by the script.$_SESSION
: A variable that contains session data that is stored on the server.$_REQUEST
: A variable that contains all of the data that is passed to the script through the URL$_ENV
: A variable that contains environment variables that are set by the operating system.
The $GLOBALS variable is a powerful tool that can be used to access global variables from anywhere in your PHP code.
<?php
$message = "Hello world!";
function printMessage (){
echo $GLOBALS['message'];
}
printMessage(); // output: Hello world!
?>
The safer way to access global variables inside a function is to use the global keyword global $message;
The $_SERVER superglobal variable is an associative array that contains information about the server environment.
Here are some of the elements of the $_SERVER superglobal variable:
<?php
echo $_SERVER['PHP_SELF']; //This returns the file name of the script that is currently being executed.
echo "<br>";
echo $_SERVER['SERVER_NAME']; //This returns the name of the server that is hosting the site.
echo "<br>";
echo $_SERVER['HTTP_HOST']; //This returns the hostname of the client that is making the request.
echo "<br>";
echo $_SERVER['HTTP_REFERER']; //This returns the URL of the page that the client was on before they came to the current page.
echo "<br>";
echo $_SERVER['HTTP_USER_AGENT']; //This returns the user agent of the client that is making the request.
echo "<br>";
echo $_SERVER['SCRIPT_NAME']; //This returns the HTTP request method that was used to make the request.
echo "<br>"
?>
We will explain more about the rest of the Superglobal variables in upcoming tutorials.
That’s all for now, I hope that was useful information for you, thank you for reading.