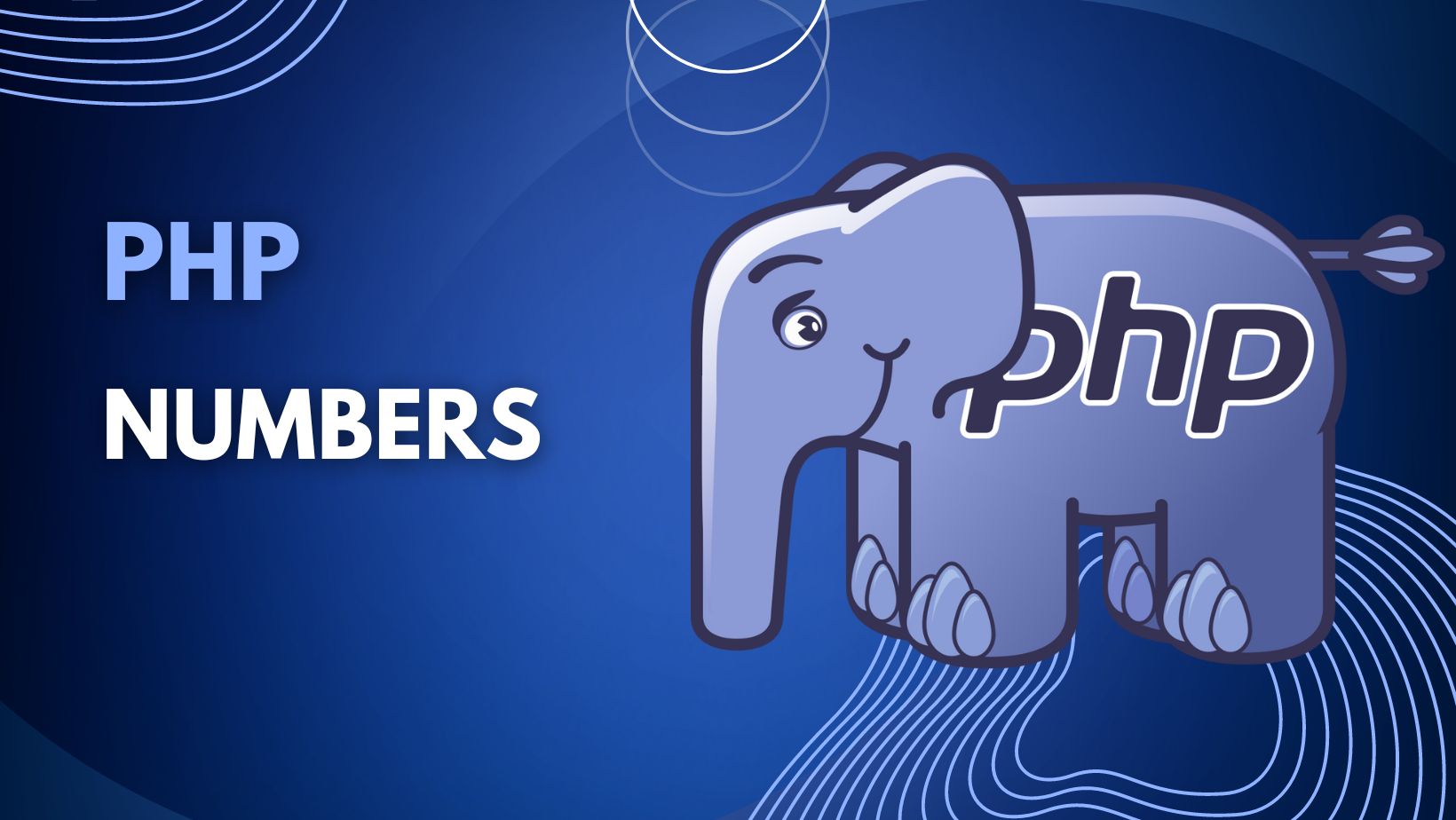
Learn everything about PHP numbers, Integer, and float and the essential functions to deal with them.
Cool Things To Do With The PHP String Functions
PHP supports two types of numbers: integers and floats numbers.
Integers are whole numbers without decimals. They can be positive, negative, or zero. The value can be between -2147483648 and 2147483647 in 32 bit systems, and between -9223372036854775808 and 9223372036854775807 in 64 bit systems, The maximum value of an integer depends on the PHP version and the system architecture.
Floats are numbers with decimals. They can be positive, negative, or zero. Floating-point numbers are stored as a decimal number with a decimal point and an exponent. The exponent tells how many places to the right of the decimal point the number is. The size of a float is platform-dependent, although a maximum of approximately 1.8e308 with a precision of roughly 14 decimal digits is a common value (the 64 bit IEEE format).
<?php
$integerNumber = 15; //integer
$floatNumber = 15.63 //float
?>
If you want to know the maximum and minimum supported. PHP has some predefined constants to let you know:
- PHP_INT_MAX – The largest integer supported.
- PHP_INT_MIN – The smallest integer supported.
- PHP_FLOAT_MAX – The largest floating point number supported.
- PHP_FLOAT_MIN – The smallest positive floating point number supported.
PHP Integers
Here are some built-in integer functions that are very important to know.
Make It Integer
is_int() You can use this function to make sure that the number value is an integer because sometimes the value comes as a string. and to convert the value to an integer we use intval().
<?php
$number = "15"; //this is string because ""
if(is_int($number)){
echo 'ok <br>';
}else{
echo 'the number needs to be converted <br>';
$toInt = intval($number);
if(is_int($toInt)){
echo 'the number converted to integer <br>';
}
}
/*
output:
the number needs to be converted
the number converted to integer
*/
?>
PHP converts string numbers to numbers when they are used in mathematical operations.
<?php
$x = "15"; //this is string because ""
$y = 5;
echo $x + $y;
// output 20
$x = "2.5"; //this is string because ""
$y = 2;
echo $x * $y;
// output 5
?>
Make It Floating-point
You can use is_float() or is_double() functions to make sure that the number value is a float because sometimes the value comes as a string or integer. and to convert the value to float we use floatval().
<?php
$number = "15.65"; //this is string because ""
if(is_float($number)){
echo 'ok <br>';
}else{
echo 'the number needs to be converted <br>';
$toInt = floatval($number);
if(is_float($toInt)){
echo 'the number converted to float <br>';
}
}
/*
output:
the number needs to be converted
the number converted to float
*/
?>
Essential Functions
I have collected some useful PHP number functions that I use in every project.
number_format()
number_format() is one of the most used functions especially with money, the function Formats a number and optionally decimal digits.
<?php
$number = 115.789569;
$formatted_number = number_format($number, 2);
echo $formatted_number; // 115.79
?>
rand()
You can generate a random integer between minimum and maximum values or just any number.
<?php
echo rand() .'<br>';
echo rand(1,10) .'<br>' ;//get a random integer between 0 and 10
?>
is_numeric()
The is_numeric()
function in PHP can be used to check if a variable is a number, including numeric strings. It returns true
if the variable is numeric, and false
if it is not.
<?php
$x = "5985";
$y = 5985;
$z = 'hello';
echo is_numeric($x)? 'numeric' :'not a number';
echo '<br>';
echo is_numeric($y)? 'numeric' :'not a number';
echo '<br>';
echo is_numeric($z)? 'numeric' :'not a number';
?>
We used the Elvis operator, ?:
to print “numeric “or “not a number” depending on the return of is_numeric.
That’s all for now, I hope that was useful information for you.