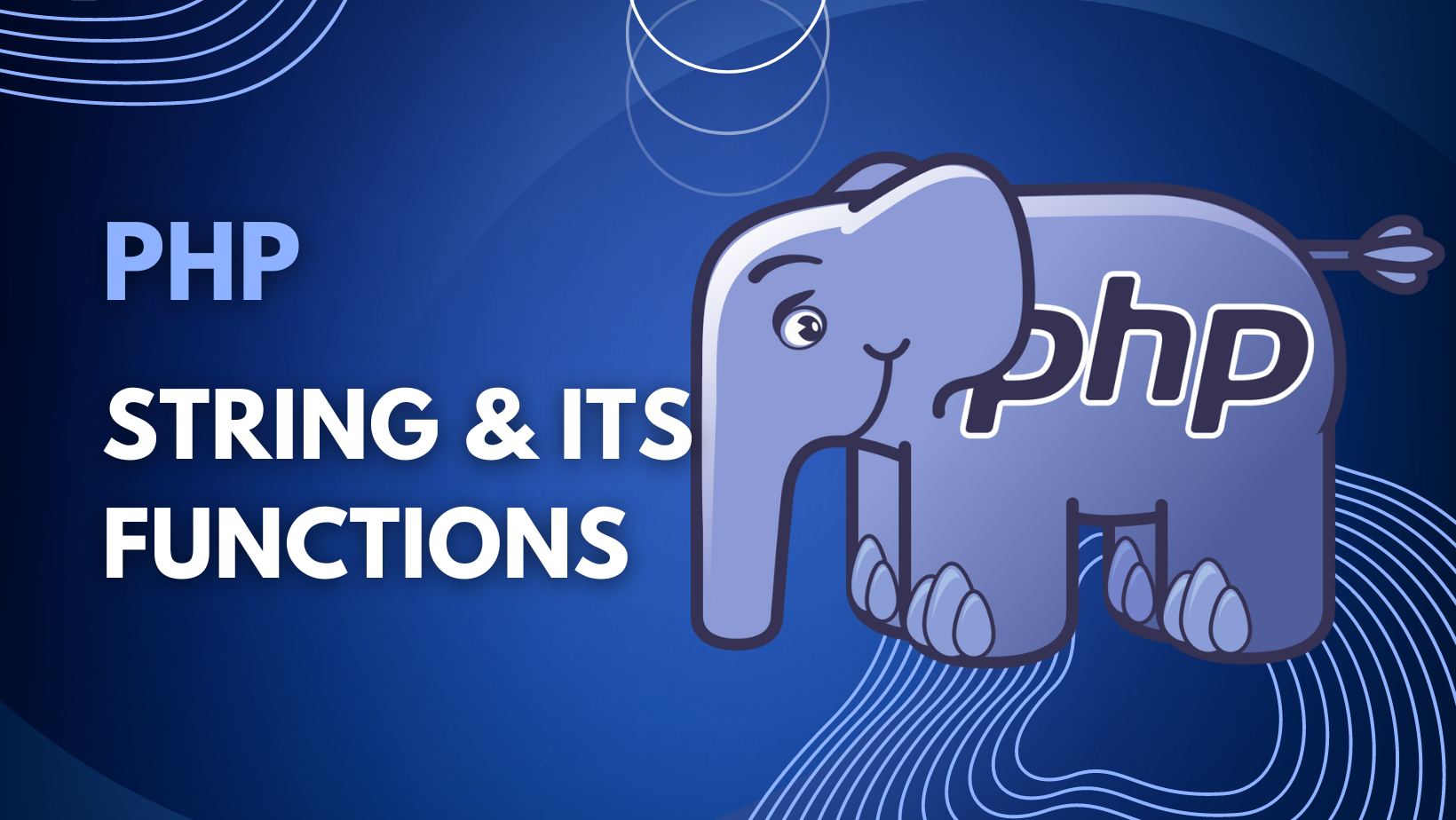
This Tutorial will cover the most used PHP functions that work with the string data type such as displaying only part of a text, removing HTML tags to get the text only, converting text to upper case and lower case, removing all whitespace from a string, and more.
Learn more about PHP:
Is PHP Dead? Why PHP? Is PHP a popular language?
Everything About PHP Variables | Learn PHP #01
PHP String
PHP strings are one of the PHP data types, String is like text. It is a sequence of characters, like “Hello world!”, And can be manipulated using a variety of string functions.
There are three ways to specify a string in PHP:
The simplest way to specify a string is to enclose it in single quotes.
$message = 'Hello world!';
Another way to specify a string is to enclose it in double quotes.
$string = "Hello world!";
The third way is useful for specifying long strings or strings that contain special characters.
$string = <<<EOF
Imagine a long string that spans multiple lines.
Some lines contains some special characters, such as a newline (\n) and a tab (\t).
EOF;
Anyways most of the time we use method one or two.
PHP String Functions
PHP strings can be manipulated using a variety of string functions. Some of the most common string functions include:
strval()
strval()
to convert any value to a string for example let’s convert a number variable to a string. we can use is_string()
to check the value if it’s a string.
<?php
$x = 256;
echo is_string($x) .'<br>'; // output nothing
$sc = strval($x); // convert to string
echo is_string($sc) .'<br>'; // output 1
?>
trim()
trim()
function is very useful for removing whitespace from the beginning and end of a string, you can use it with submitted form data such as login and registration.
<?php
$string = ' myemail@coolmail.com ';
echo trim($string);
//output: myemail@coolmail.com
?>
substr()
This awesome function returns a substring of a string, we can use it to make a short description from a long text. substr(STRING,WHER TO START, WHERE TO END)
<?php
$string = 'PHP strings can be manipulated using a variety of string functions. Some of the most common string functions';
echo substr($string,0,37).'...';
//output: PHP strings can be manipulated using ...
?>
strip_tags()
What if you got the text with HTML tags, that happens a lot with blog posts that are made with WYSIWYG HTML Editor, so we can use strip_tags()
to strip HTML tags and get the text only.
<?php
echo strip_tags("<small>Hello</small> <b>world!</b>");
//output: Hello world!
?>
str_replace()
str_replace()
is the function for replacing some characters with some other characters in a string. let’s replace “Hello” with “Hi” in this text “Hello, world!, Hello, people!”.
<?php
$string = "Hello, world!, Hello, people!";
echo str_replace("Hello","Hi",$string);
//output: Hi, world!, Hi, people!
?>
strlen()
we use strlen() to get the length of a string, let’s say you want to make a limit to an input like twitter or X. so strlen() will be a good help.
<?php
$string = "Hello, world!, Hello, people!";
echo strlen($string);
//output: 29
?>
str_word_count()
What if you want to count the words of an essay, str_word_count() will help you to get the words count in a string.
<?php
$string = "Hello, world!, Hello, people!";
echo str_word_count($string);
//output: 4
?>
strpos()
To search a world in a string you can use strpos(), it returns the position of the first occurrence of a substring in a string.
<?php
$string = "Hello, world!, Hello, people!";
echo strpos($string, "world");
//output: 7
?>
Some more functions
- strtoupper(): Converts a string to uppercase.
- strtolower(): Converts a string to lowercase.
- strrev(): Reverses the order of the characters in a string.
Conclusion
We have explained the PHP string data type what you can do with it. PHP has so many built in functions to deal with strings that can save time of coding. Here we covered the most used functions but you can find more in PHP documentation.
Essential Functions To Deal With Numbers in PHP | Learn PHP #03