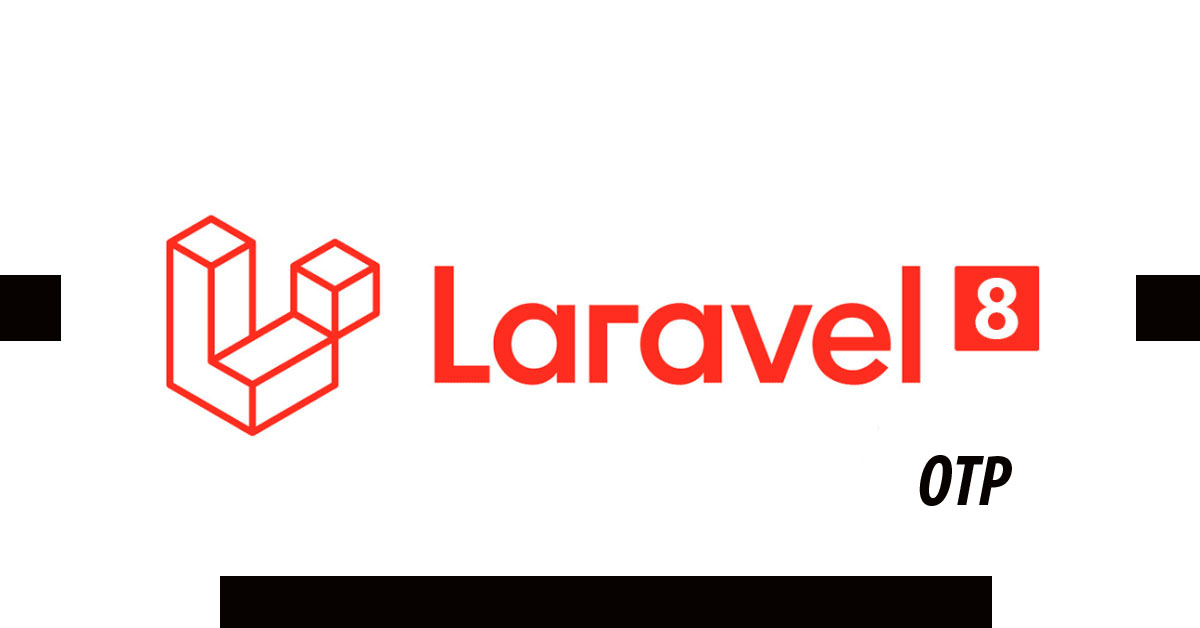
If you want to send your end-user 4 to 6 digits codes to verify their mobile number or verify an action such as resetting a password, log in via mobile or email. Here you will learn verification via OTP in Laravel.
The power of the one-time password is the simplicity, I send the users a small code, and voila! they are on my system.
What you will learn
- Installing OTP package in laravel
- Generating OTP
- Validating OTP
Installing OTP package in laravel
I have searched for a simple package that gets the job done and I found ichtrojan/laravel-otp, it’s a simple package to generate and validate OTPs.
We will install it via composer
composer require ichtrojan/laravel-otp
Add service provider and alias to the config/app.php file
'providers' => [
...
Ichtrojan\Otp\OtpServiceProvider::class,
];
//
'aliases' => [
...
'Otp' => Ichtrojan\Otp\Otp::class,
];
Finally, we need to run a migration
php artisan migrate
Generating OTP
To generate OTP you need three parameters:
Otp::generate($identifier,$digits = 4, int $validity = 15)
The first: identifier, can be user id, mobile number, email anything to know who owns this OTP.
The second: digit, the number of the digit in the OTP the default is 4 and max is 6, it’s an optional parameter.
The third: validity, the validity period in minutes it’s optional, the default is 15 minutes.
The package success response will be
{
"status": true,
"token": "7881",
"message": "OTP generated"
}
Let create a function inside a controller for generating OTP
use Otp;
class LoginController extends Controller
{
private $otp;
public function __construct(Otp $Otp)
{
$this->otp = $Otp;
}
public function GetloginOtp(Request $request)
{
/* generate otp */
$otp = $this->otp->generate($request->mobile, 6, 15);
/* you and send OTP via sms or email */
$smsOrEmailMessage = 'Use this code for login': '.$otp->token;
/* json response */
return response([
'success'=> $otp->status,
'message' => $otp->message
]);
}
}
Validating OTP
To validate the OTP code we need to use this function
Otp::validate($identifier,$token)
The possible responses will be
On success
{
"status": true,
"message": "OTP is valid"
}
On fail
{
"status": false,
"message": "OTP does not exist"
}
/****/
{
"status": false,
"message": "OTP is not valid"
}
/****/
{
"status": false,
"message": "OTP Expired"
}
Let’s add the below function our controller for validating OTP
public function loginOtp(Request $request)
{
/* validate otp */
$otp = $this->otp->validate($request->mobile,$request->otp);
if($otp->status){
// add your action code here
}
/* json response */
return response([
'success'=> $otp->status,
'message' => $otp->message
]);
}
I hope, I could help! Thanks.