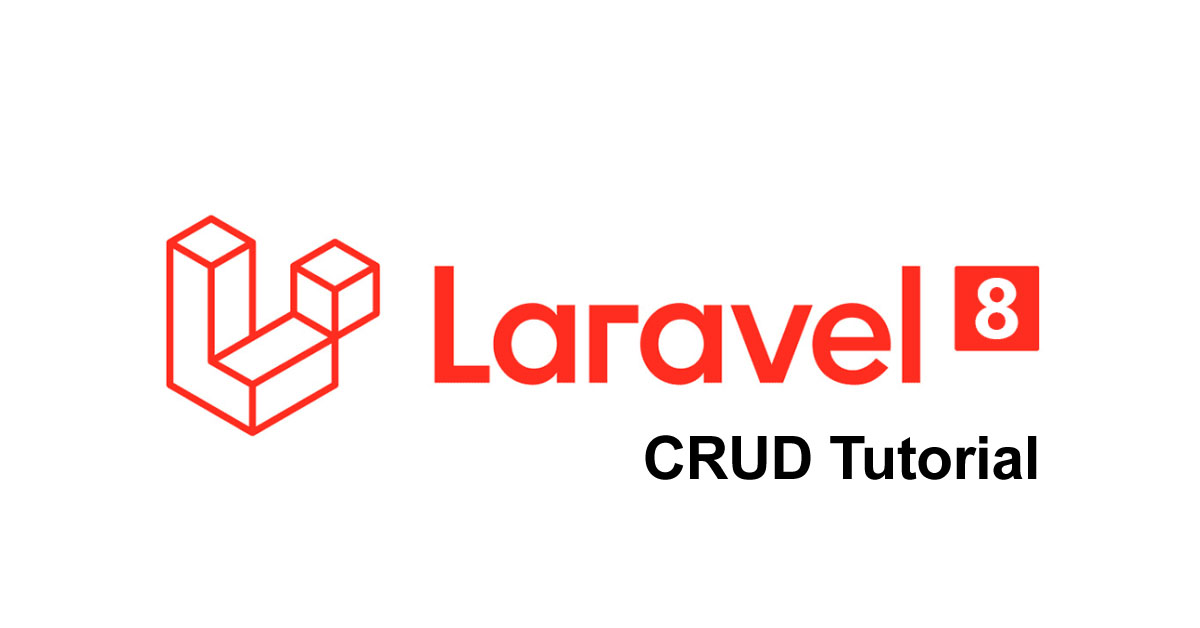
In this tutorial, I’m going to explain to you how to create a sample CRUD Laravel web application with code example from the real world for inserting, editing, showing, and delete data from the database that will put you in the start position. we use Laravel 8, so let get started.
What you will learn in this tutorial.
- Laravel app installation.
- Database configuration.
- Creating tables and migration.
- Creating Controller and model.
- Adding routes.
- Creating views.
Laravel app installation.
We will create a Laravel application via Composer, you should have PHP and Composer installed.
composer create-project laravel/laravel crud-app
cd example-app
we will run Laravel’s local development server using the Artisan CLI command for making sure that the installation went right, you should see the Laravel welcome page.
php artisan serve
Database configuration.
I’m using Xampp as a local server. I have created a database with the name “crud-app” in phpMyAdmin. In the .env file, we will configure our new database connection.
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=crud-app
DB_USERNAME=root
DB_PASSWORD=
Creating tables and migration.
We will create a migration file for creating the “posts” table in the database, we will use the below artisan command and make user you are in the root directory of the crud-app project.
php artisan make:migration create_post_table --create=posts
then open our new file in “database\migrations”, its name will be a date following by create_post_table.php for example “2021_08_02_084303_create_post_table.php”.
Add the following changes to the file.
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class CreatePostTable extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('posts', function (Blueprint $table) {
$table->id();
$table->string('title');
$table->text('description');
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropIfExists('posts');
}
}
Let’s add our table to the database via this artisan command.
php artisan migrate
Creating Controller and model.
Our database is ready now, so we need to create “PostController” and “Post” model for Adding, Editing, Showing, and Deleting posts. Let’s do this.
php artisan make:controller PostController --resource --model=Post
Open the “app\Http\Controllers\PostController.php” file and make the following changes.
<?php
namespace App\Http\Controllers;
use App\Models\Post;
use Illuminate\Http\Request;
class PostController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
$posts = Post::latest()->get();
return view('posts.index',compact('posts'));
}
/**
* Show the form for creating a new resource.
*
* @return \Illuminate\Http\Response
*/
public function create()
{
return view('posts.create');
}
/**
* Store a newly created resource in storage.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\Response
*/
public function store(Request $request)
{
$request->validate([
'title' => 'required',
'description' => 'required',
]);
Post::create($request->all());
return redirect()->route('posts.index')
->with('success','Post created successfully.');
}
/**
* Display the specified resource.
*
* @param \App\Models\Post $post
* @return \Illuminate\Http\Response
*/
public function show(Post $post)
{
return view('posts.show',compact('post'));
}
/**
* Show the form for editing the specified resource.
*
* @param \App\Models\Post $post
* @return \Illuminate\Http\Response
*/
public function edit(Post $post)
{
return view('posts.edit',compact('post'));
}
/**
* Update the specified resource in storage.
*
* @param \Illuminate\Http\Request $request
* @param \App\Models\Post $post
* @return \Illuminate\Http\Response
*/
public function update(Request $request, Post $post)
{
$request->validate([
'title' => 'required',
'description' => 'required',
]);
$post->update($request->all());
return redirect()->route('posts.index')
->with('success','Post updated successfully');
}
/**
* Remove the specified resource from storage.
*
* @param \App\Models\Post $post
* @return \Illuminate\Http\Response
*/
public function destroy(Post $post)
{
$post->delete();
return redirect()->route('posts.index')
->with('success','Post deleted successfully');
}
}
Then open the “app\model\Post.php” file. and make it looks like this.
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
class Post extends Model
{
use HasFactory;
protected $fillable = [
'title', 'description'
];
}
Adding routes.
We need to create the links that the users will use for navigating your crud-app. Laravel made it easy for us, open “app\routes\web.php” and make the following changes in the code.
<?php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\PostController;
/*
|--------------------------------------------------------------------------
| Web Routes
|--------------------------------------------------------------------------
|
| Here is where you can register web routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| contains the "web" middleware group. Now create something great!
|
*/
Route::resource('posts', PostController::class);
Route::get('/', function () {
return view('welcome');
});
Creating views
We will create the web pages for the user interface that will be loading on the web browser, we are going to use HTML and bootstrap. we will create these blade file in the “resources\views” folders.
layout\layout.blade.php
<!DOCTYPE html>
<html>
<head>
<title>Laravel CRUD-Application - ahmedshaltout.com</title>
<link href="https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/4.0.0-alpha/css/bootstrap.css" rel="stylesheet">
</head>
<body>
<div class="container">
@yield('content')
</div>
</body>
</html>
posts\index.blade.php
@extends('layout.layout')
@section('content')
<div class="row" style="margin-top: 5rem;">
<div class="col-lg-12 margin-tb">
<div class="pull-left">
<h2>Posts</h2>
</div>
<div class="pull-right">
<a class="btn btn-success" href="{{ route('posts.create') }}"> Create New Post</a>
</div>
</div>
</div>
@if ($message = Session::get('success'))
<div class="alert alert-success">
<p>{{ $message }}</p>
</div>
@endif
<table class="table table-bordered">
<tr>
<th>ID</th>
<th>Title</th>
<th>description</th>
<th width="280px">Action</th>
</tr>
@foreach ($posts as $post)
<tr>
<td>{{ $post->id }}</td>
<td>{{ $post->title }}</td>
<td>{{ \Str::limit($post->description, 90) }}</td>
<td>
<form action="{{ route('posts.destroy',$post->id) }}" method="POST">
<a class="btn btn-info" href="{{ route('posts.show',$post->id) }}">Show</a>
<a class="btn btn-primary" href="{{ route('posts.edit',$post->id) }}">Edit</a>
@csrf
@method('DELETE')
<button type="submit" class="btn btn-danger">Delete</button>
</form>
</td>
</tr>
@endforeach
</table>
@endsection
posts\create.blade.php
@extends('layout.layout')
@section('content')
<div class="row">
<div class="col-lg-12 margin-tb">
<div class="pull-left">
<h2>Add New Post</h2>
</div>
<div class="pull-right">
<a class="btn btn-primary" href="{{ route('posts.index') }}"> Back</a>
</div>
</div>
</div>
@if ($errors->any())
<div class="alert alert-danger">
<strong>Whoops!</strong> There were some problems with your input.<br><br>
<ul>
@foreach ($errors->all() as $error)
<li>{{ $error }}</li>
@endforeach
</ul>
</div>
@endif
<form action="{{ route('posts.store') }}" method="POST">
@csrf
<div class="row">
<div class="col-xs-12 col-sm-12 col-md-12">
<div class="form-group">
<strong>Title:</strong>
<input type="text" name="title" class="form-control" placeholder="Enter Title">
</div>
</div>
<div class="col-xs-12 col-sm-12 col-md-12">
<div class="form-group">
<strong>Description:</strong>
<textarea class="form-control" style="height:150px" name="description" placeholder="Enter Description"></textarea>
</div>
</div>
<div class="col-xs-12 col-sm-12 col-md-12 text-center">
<button type="submit" class="btn btn-primary">Submit</button>
</div>
</div>
</form>
@endsection
posts\edit.blade.php
@extends('layout.layout')
@section('content')
<div class="row">
<div class="col-lg-12 margin-tb">
<div class="pull-left">
<h2>Edit Product</h2>
</div>
<div class="pull-right">
<a class="btn btn-primary" href="{{ route('posts.index') }}"> Back</a>
</div>
</div>
</div>
@if ($errors->any())
<div class="alert alert-danger">
<strong>Whoops!</strong> There were some problems with your input.<br><br>
<ul>
@foreach ($errors->all() as $error)
<li>{{ $error }}</li>
@endforeach
</ul>
</div>
@endif
<form action="{{ route('posts.update',$post->id) }}" method="POST">
@csrf
@method('PUT')
<div class="row">
<div class="col-xs-12 col-sm-12 col-md-12">
<div class="form-group">
<strong>Title:</strong>
<input type="text" name="title" value="{{ $post->title }}" class="form-control" placeholder="Title">
</div>
</div>
<div class="col-xs-12 col-sm-12 col-md-12">
<div class="form-group">
<strong>Description:</strong>
<textarea class="form-control" style="height:150px" name="description" placeholder="Detail">{{ $post->description }}</textarea>
</div>
</div>
<div class="col-xs-12 col-sm-12 col-md-12 text-center">
<button type="submit" class="btn btn-primary">Submit</button>
</div>
</div>
</form>
@endsection
posts\show.blade.php
@extends('layout.layout')
@section('content')
<div class="row">
<div class="col-lg-12 margin-tb">
<div class="pull-left">
<h2> Show Post</h2>
</div>
<div class="pull-right">
<a class="btn btn-primary" href="{{ route('posts.index') }}"> Back</a>
</div>
</div>
</div>
<div class="row">
<div class="col-xs-12 col-sm-12 col-md-12">
<div class="form-group">
<strong>Title:</strong>
{{ $post->title }}
</div>
</div>
<div class="col-xs-12 col-sm-12 col-md-12">
<div class="form-group">
<strong>Description:</strong>
{{ $post->description }}
</div>
</div>
</div>
@endsection
Finally, test the app.
php artisan serve
and open the browser and go to http://127.0.0.1:8000/posts.
You can download the code from GitHub
https://github.com/migofk/Laravel-8-CRUD
I hope I could help you, thanks.