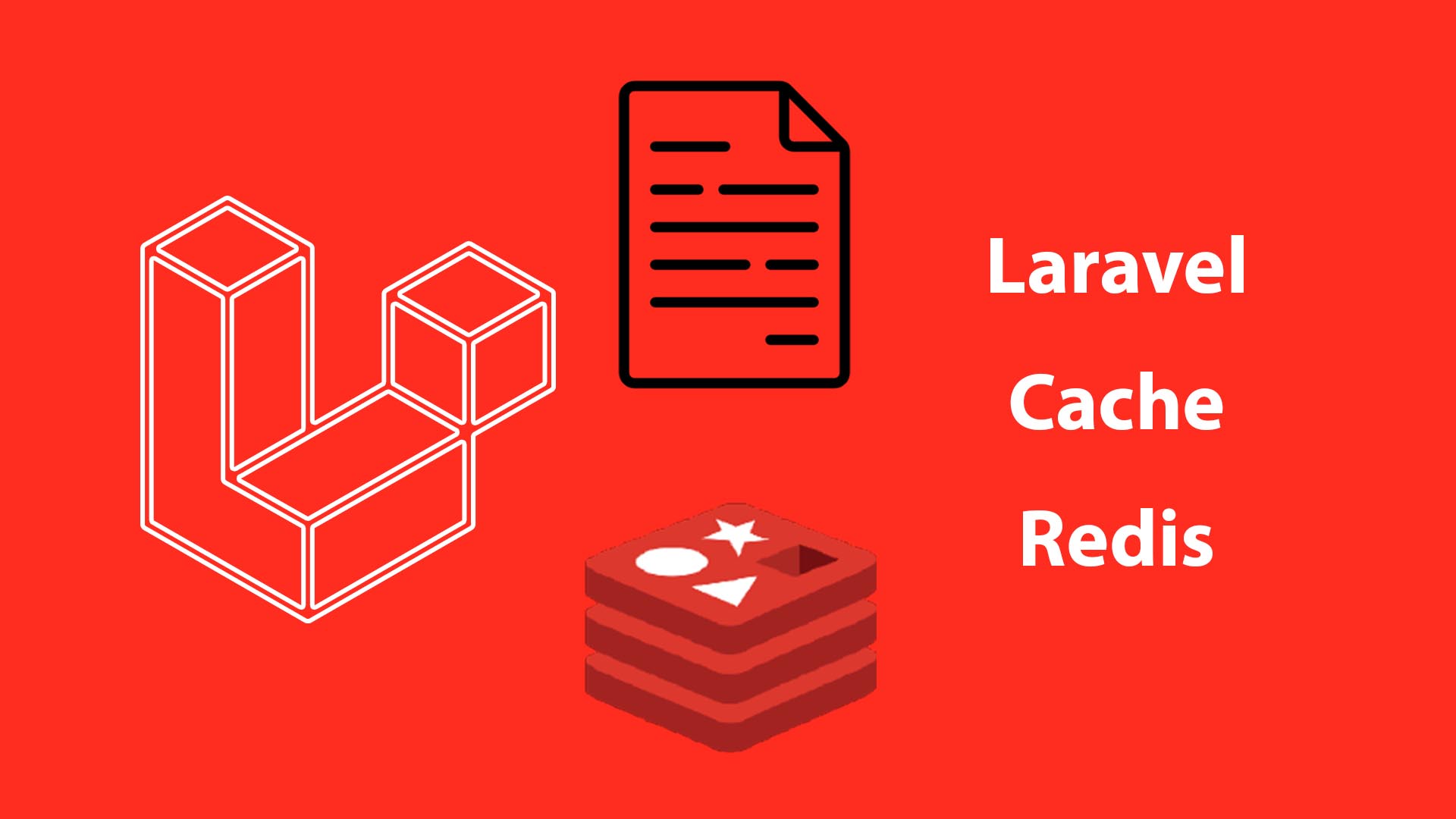
Let’s create a Laravel cache class to store data in a Redis server or files. Laravel has powerful APIs for caching so let’s get started.
If you like to watch this tutorial as a youtube video on my channel click here
Why should we use Laravel Cache?
Some tasks in your application could take some time to complete or are CPU intensive. For example data retrieving from a database or processing some data before the response. So these tasks will make your application slow and may cost more money for hosting. So to avoid those problems, Here comes Laravel cache API, we only save the result of these heavy tasks in files, in-memory data stores, or even databases. And Laravel supports various cache services with fast data retrieval that can speed up our applications.
Understanding cache
Let’s say we query the database to retrieve product data by id. after getting these data we can cache the data in a cache store. so next time we get the product data, we get it from the cache store. The data in the cache store is similar to an Object with kay and value {key: value}
, We give it a unique key and assign the value to that key. the key must be unique otherwise we will override the existing one. We retrieve the data from that cache by the key.
//store data
Cache::put('key', 'value');
Cache::put('product_'.$id, $product);
//retrieve data
$value = Cache::get('key');
$product= Cache::get(product_'.$id);
Configuring cache driver
In .env
file, we have CACHE_DRIVER
variable to set the default cache driver by default file. We can configure our cache stores in config\cache.php
at stores array. In this example, we will use file and Redis.
File
Laravel stores the cached data in files on the local disk and uses its file system to manage it, the path for saving the cache framework/cache/data
, it’s cheap no requirements nor a new host is needed. it’s slower than Redis but with big data such as articles, they do mostly the same. it’s suitable for small and medium traffic. It works with high traffic as well but for better results use Redis.
Redis
Redis is an in-memory data structure store, used as a distributed, in-memory key–value database, cache and message broker. You need to install Redis on your machine first before we start, you can find the instructors here in Redis doc or you can skip this part if you want to use files
store only.
Let’s install predis/predis package, the flexible and feature-complete Redis client for PHP and Laravel.
composer require predis/predis
And make sure the configuration for the Redis client is 'predis'
in config\database.php
. I’m using Windows 10 with WSL2 and Ubuntu 22, that’s my configuration.
'redis' => [
'client' => env('REDIS_CLIENT', 'predis'),
'options' => [
'cluster' => env('REDIS_CLUSTER', 'redis'),
'prefix' => env('REDIS_PREFIX', Str::slug(env('APP_NAME', 'laravel'), '_').'_database_'),
],
'default' => [
'url' => env('REDIS_URL'),
'host' => env('REDIS_HOST', '127.0.0.1'),
'username' => env('REDIS_USERNAME'),
'password' => env('REDIS_PASSWORD'),
'port' => env('REDIS_PORT', '6379'),
'database' => env('REDIS_DB', '0'),
],
'cache' => [
'url' => env('REDIS_URL'),
'host' => env('REDIS_HOST', '127.0.0.1'),
'username' => env('REDIS_USERNAME'),
'password' => env('REDIS_PASSWORD'),
'port' => env('REDIS_PORT', '6379'),
'database' => env('REDIS_CACHE_DB', '1'),
],
],
Laravel cache helper class
Let’s create our cache helper that can use more than one store and we will use it in the controller later. Create a new folder and file app\Helpers\Cacher.php
<?php
namespace App\Helpers;
use Illuminate\Support\Facades\Cache;
class Cacher{
public function __construct( public string $store = 'file'){}
//file //redis
public function setCached($key,$value){
$cachedData = Cache::store($this->store)->put($key,$value);
}
public function getCached($key){
$cachedData = Cache::store($this->store)->get($key);
if($cachedData){
return json_decode($cachedData);
}
}
public function removeCached($key){
Cache::store($this->store)->forget($key);
}
}
The code explanation:
- In the constructor we have
$store
to select the cache store, here we usefile
orredis
. setCached
to store the data in the cache store with key and value.getCached
to retrieve the data by key.removeCached
to remove the data from the cache store.
Using Cacher class with controllers
<?php
namespace App\Http\Controllers\Admin;
//
use App\Helpers\Cacher;
class ProductController extends Controller
{
//we call the cacher with the store name file or redis
private $cacher;
public function __construct(){
$this->cacher = new Cacher('file');
}
/*******************************************/
public function store(ProductStoreRequest $request)
{
$data = $request->validated();
$product = new ProductResource(Product::create($data));
$this->cacher->setCached('product_'.$product->id, $product->toJson());
return response()->json($product, 200);
}
/*******************************************/
public function show(Request $request, $id)
{
$cachedData = $this->cacher->getCached('product_'.$id);
if($cachedData){
$product = $cachedData;
}else{
$product = new ProductResource(Product::find($id));
$this->cacher->setCached('product_'.$id, $product->toJson());
}
return response()->json($product, 200);
}
/*******************************************/
public function update(ProductUpdateRequest $request, Product $product)
{
$data = $request->validated();
$product->update($data);
$product->refresh();
$success['data'] = new ProductResource($product);
$this->cacher->setCached('product_'.$product->id, $success['data']->toJson());
$success['success'] = true;
return response()->json($success, 200);
}
/*******************************************/
public function destroy(ProductDeleteRequest $request,Product $product)
{
$this->cacher->removeCached('product_'.$product->id);
$product->delete();
}
}
In the constructor we select file
to be our cache driver, you can change it to redis
if you don’t want to use file
. and It will work.
In the above example, we used our cache class in most cases. you don’t have to use API resources new ProductResource($product);
when saving the data, you can use the collection directly.
When we store a new product we create its cache instance, with the update we override the existing one. with the show function, we check if there is a cached version, If not found, we get it from the database and then create a new cache for it.
One thought on “Laravel Cache Redis And File Store Tutorial With Example.”