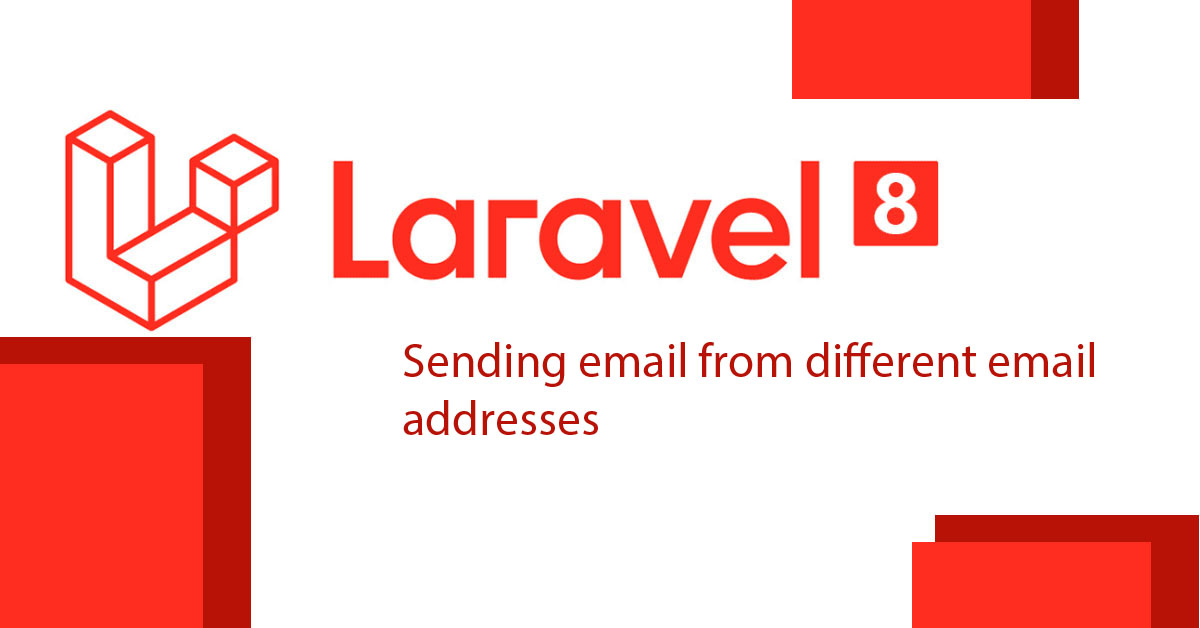
You can configure multiple emails in Laravel to send notifications from, let’s say you need to send welcome notification from no-reply@example.com and new order notification from orders@example.com, If that is what you want to do so this lesson is here for you.
What you will learn
- How to configure multiple emails in laravel
- Sending email via mailable and mailer
How to configure multiple emails in laravel
After you get your emails configuration data from cPanel or any other provider, what you will need is (Host, port, username, password, encryption).
The main email in Laravel will be in .env file and the other emails will be in mail.php.
Let’s configure our main email open .env file modify the email setting:
MAIL_MAILER=smtp
MAIL_HOST=smtp.example.com
MAIL_PORT=587
MAIL_USERNAME=no-reply@example.com
MAIL_PASSWORD=password
MAIL_ENCRYPTION=tls
MAIL_FROM_ADDRESS=no-reply@example.com
MAIL_FROM_NAME="${APP_NAME}"
Make sure the ‘from’ email address is the same as the sender’s email address.
We need to add the other email let’s open the config\mail.php file and add our emails in the ‘mailers’
'default' => env('MAIL_MAILER', 'smtp'),
'mailers' => [
'smtp' => [
'transport' => 'smtp',
'host' => env('MAIL_HOST', 'smtp.mailgun.org'),
'port' => env('MAIL_PORT', 587),
'encryption' => env('MAIL_ENCRYPTION', 'tls'),
'username' => env('MAIL_USERNAME'),
'password' => env('MAIL_PASSWORD'),
'timeout' => null,
'auth_mode' => null,
],
'order' => [
'transport' => 'smtp',
'host' => env('MAIL_HOST', 'smtp.mailgun.org'),
'port' => env('MAIL_PORT', 587),
'encryption' => env('MAIL_ENCRYPTION', 'tls'),
'username' => 'order@example.com',
'password' => env('MAIL_PASSWORD'),
'timeout' => null,
'auth_mode' => null,
],
'booking' => [
'transport' => 'smtp',
'host' => env('MAIL_HOST', 'smtp.mailgun.org'),
'port' => env('MAIL_PORT', 587),
'encryption' => env('MAIL_ENCRYPTION', 'tls'),
'username' => 'booking@example.com',
'password' => env('MAIL_PASSWORD'),
'timeout' => null,
'auth_mode' => null,
],
///
You can The ‘default’ in mail.php for the main email for your application anytime.
Sending email via mailable and mailer
We will create a class the will send emails for us and we will call it in the controller. the parameters will be the username, message, subject, the email address we send from.
Let’s generate a mailable class via this command:
php artisan make:mail GeneralEmail
It will generate the class for us in app\Mail\GeneralEmail.php, open this file and modify it as the following
<?php
namespace App\Mail;
use Illuminate\Bus\Queueable;
use Illuminate\Contracts\Queue\ShouldQueue;
use Illuminate\Mail\Mailable;
use Illuminate\Queue\SerializesModels;
class GeneralEmail extends Mailable
{
use Queueable, SerializesModels;
public $name;
public $message;
public $subject;
public $fromEmail;
public $fromName;
/**
* Create a new message instance.
*
* @return void
*/
public function __construct($name, $message, $subject,$fromEmail)
{
$this->name = $name;
$this->message = $message;
$this->subject = $subject;
$this->fromEmail = $fromEmail;
$this->fromName = env('MAIL_FROM_NAME');
}
/**
* Build the message.
*
* @return $this
*/
public function build()
{
return $this->from($this->fromEmail,$this->fromName)
->subject($this->subject)
->markdown('mail.general-email')
->with([
'userName' => $this->name,
'themessage' => $this->message,
]);;
}
}
we need to create the email template so we will create a new view resources\views\mail\general-email.blade.php and add the following code
<h1>Welcome: {{$userName}}</h1>
<p>{{$themessage}}</p>
The class is ready to use in your application for sending emails.
We need the route and controller for testing our email class. let’s create the controller
php artisan make:controller SendEmailController
Open app\Http\Controllers\SendEmailController.php file and create a new function
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Mail\GeneralEmail;
use Mail;
class SendEmailController extends Controller
{
public function sendemail(){
Mail::mailer('order')->to('user@example.com')->send(new GeneralEmail('User name','the message welcome'
,'the subject','order@example.com'
));
return 'sent';
}
}
In sendmail function, we use Laravel Mail class with mailer that takes the index of the mail we want to send from the mailer array in the mail.php file.
Let’s test, create a route for our controller function in web.php
use App\Http\Controllers\SendEmailController;
//
Route::get('send-email', [SendEmailController::class,'sendemail']);
//
Finally run the server and call the route in the browser it should display ‘sent’.
I hope I could help thanks.