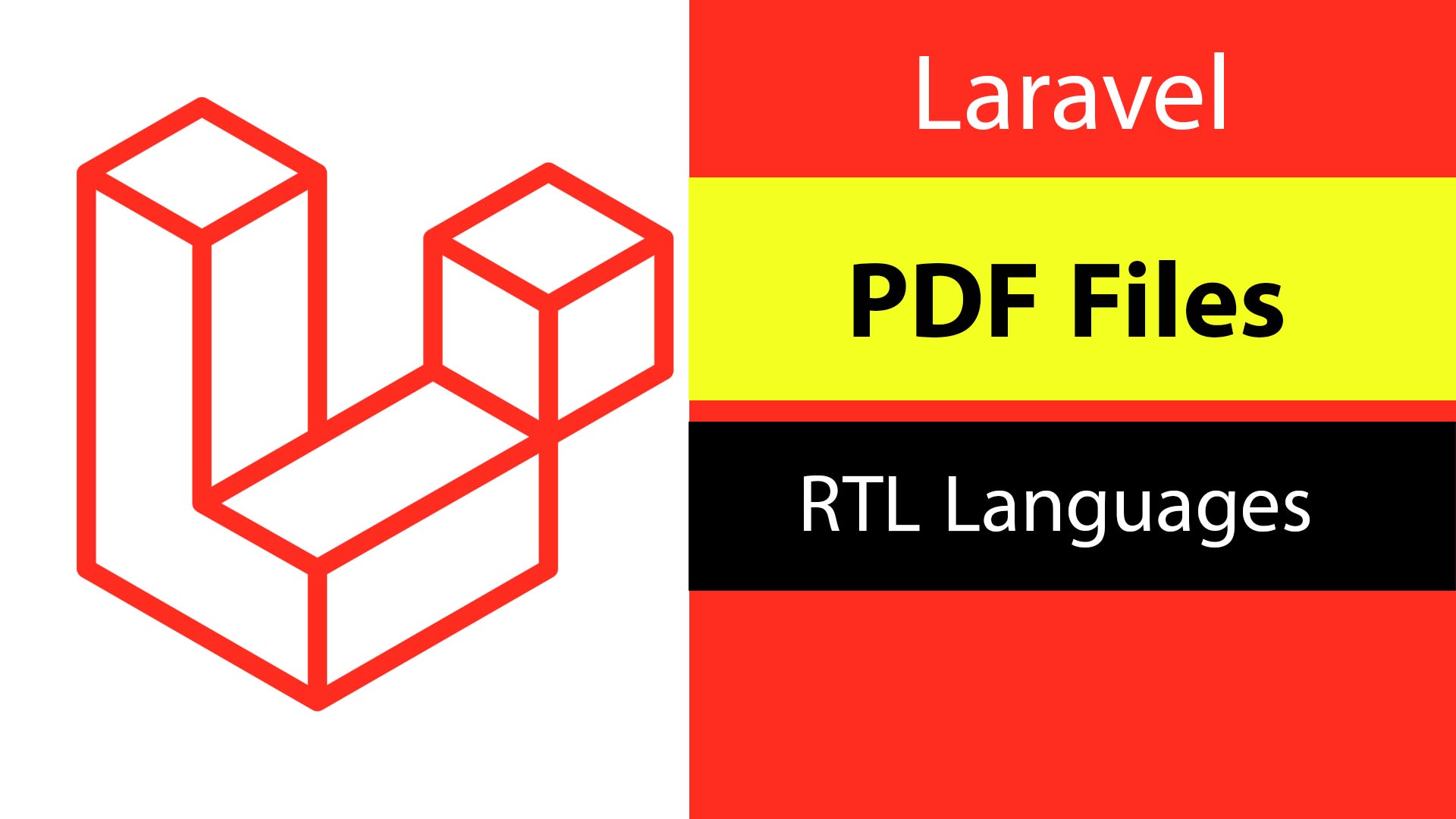
The easiest way to generate a PDF file in Laravel that can support Arabic and other RTL languages.
I had so many problems achieving that until I found niklasravnsborg/laravel-pdf package. I could solve so many problems but I needed to have a font that supports the Arabic language otherwise it becomes like this [][][][]. Thanks to Google Fonts with its variety of free fonts. That’s all I needed and I had an awesome pdf file.
Useful Tutorials:
- Laravel E-commerce Product Model With Price and Discount Concept.
- Laravel 9 Localization With Multi-languages Database Tutorial
- Building Laravel & Vue.js Chat App 00 | Introduction
Laravel PDF
Let’s install the niklasravnsborg/laravel-pdf via composer, I’m using Laravel 10.
composer require niklasravnsborg/laravel-pdf
We can add the Service Provider and the Facade to config/app.php
'providers' => [
// ...
niklasravnsborg\LaravelPdf\PdfServiceProvider::class
]
//
'aliases' => [
// ...
'PDF' => niklasravnsborg\LaravelPdf\Facades\Pdf::class
]
Let’s publish the package’s config file
php artisan vendor:publish
Select the provider number from the list.
Provider: niklasravnsborg\LaravelPdf\PdfServiceProvider .................. 9
Laravel PDF Generator
Let’s create our controller and name it PDFController
. We will add one function to generate a pdf file with words translated from English to Arabic.
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use PDF;
use Storage;
class PDFController extends Controller
{
public function generate_pdf() {
$data = [
'translation'=>[
'hello' => 'اهلا',
'welcome' => 'مرحبا',
'good' => 'جيد'
]
];
// Get the current date and time.
$dateTime = now();
// Generate a unique filename.
$fileName = $dateTime->format('YmdHis') . '_translation.pdf';
//Generate the pdf file
$pdf = PDF::loadView('pdf.translation', $data);
//return $pdf->stream( $filename);
//Save the pdf file in the public storage
$pdf->save( storage_path('app/public/'.$fileName));
//Get the file url
$urlToDownload = Storage::disk('public')->url($fileName);
return response()->json([
'success' => true,
'url' => $urlToDownload,
]);
}
}
$data
is the data that will be sent to the view. We will create a unique name for the file using the date and time $dateTime
.
You can stream the file for testing while developing it $pdf->stream( $filename);
but now we are going to save the file in the public storage and we will send the download link to the front-end via RESTful API.
Below is the PDF view for that file resources\views\pdf\translation.blade.php
It’s just an HTML with Laravel blade templates. we can design it as want.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<link rel="preconnect" href="https://fonts.googleapis.com">
<link rel="preconnect" href="https://fonts.gstatic.com" crossorigin>
<link href="https://fonts.googleapis.com/css2?family=Cairo&display=swap" rel="stylesheet">
<title>Document</title>
</head>
<body style="font-family: 'Cairo', sans-serif;">
@foreach ($translation as $key=> $value)
<p>{{$key}} : {{$value}}</p>
@endforeach
</body>
</html>
I use Cairo font from Google to display Arabic characters otherwise it will be like boxes [][][][]. so that was a good solution for me.
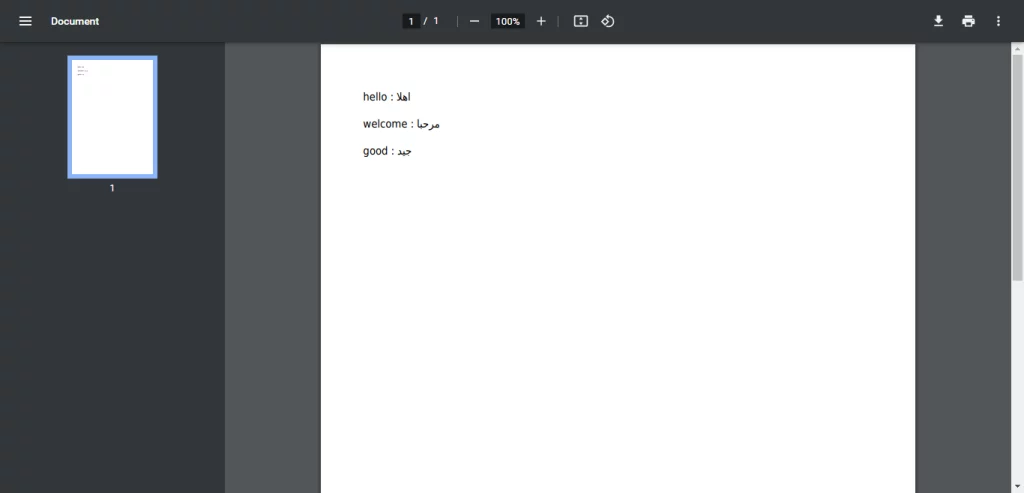
Here is the route to test the function.
use App\Http\Controllers\PDFController;
Route::get('/generate_pdf', [PDFController::class, 'generate_pdf']);
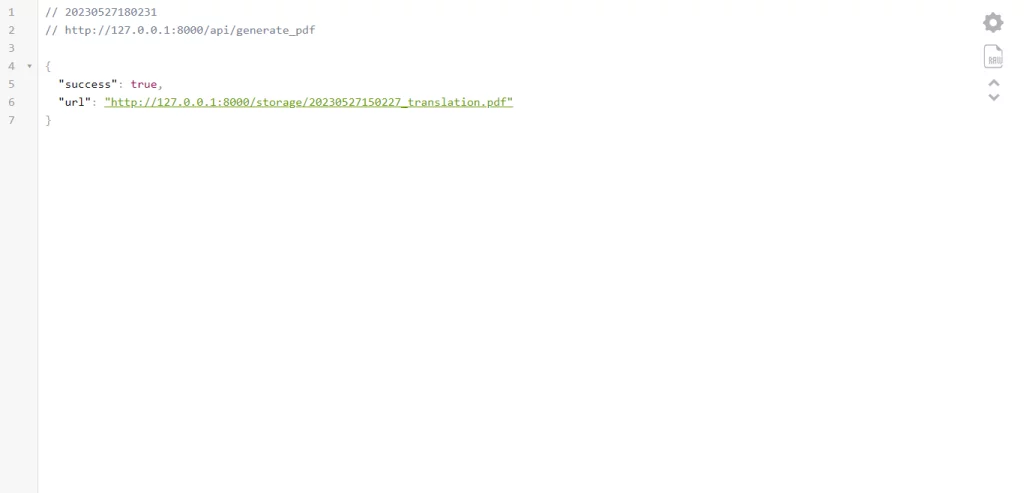
That’s all for now, I hope that was useful for you, thanks.