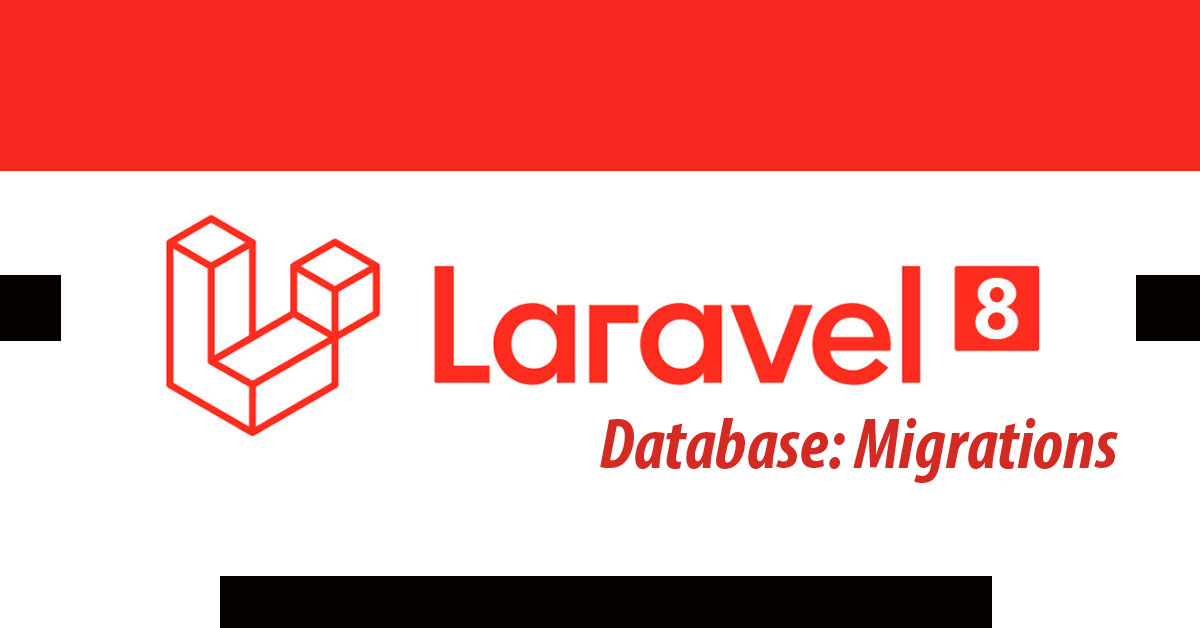
Sometimes we need to add a new column or update an existing one to an existing table in the database after the migration. it’s so easy to do, we will add a new column and rename and change the data type of another one. let’s get started.
Let’s say we have a posts table that contains id, title, and description columns
public function up()
{
Schema::create('posts', function (Blueprint $table) {
$table->id();
$table->string('title');
$table->text('description');
$table->timestamps();
});
}
We need to do three actions on that table, we need to add a new column for authors and we need to update the title to change the data type from string to text and rename the title ‘name’ to be ‘name’.
Adding a new column via laravel migration.
Let’s create the migration for adding the author column for posts
php artisan make:migration adding_author_to_posts --table=posts
In our new file in database\migrations folder add the following codes
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class AddingAuthorToPosts extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::table('posts', function (Blueprint $table) {
$table->string('author');
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::table('posts', function (Blueprint $table) {
$table->dropColumn('author');
});
}
}
And run php artisan migrate command
php artisan migrate
Now we added the new author column to our posts table.
Renaming an exsiting column via laravel migration
The next action is to rename the existing column from ‘title’ to name.
php artisan make:migration update_title_in_posts --table=posts
Let’s modify the new file in database\migrations
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class UpdateTitleInPosts extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::table('posts', function (Blueprint $table) {
$table->renameColumn('title', 'name')->change();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::table('posts', function (Blueprint $table) {
$table->dropColumn('name');
});
}
}
and run the migration
php artisan migrate
Change the data type of an existing column via laravel migration
let’s change the ‘name’ colume data type from string to text
php artisan make:migration update_name_in_posts --table=posts
In the new migration file
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class UpdateNameInPosts extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::table('posts', function (Blueprint $table) {
$table->text('name')->change();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::table('posts', function (Blueprint $table) {
$table->dropColumn('name');
});
}
}
finally, run:
php artisan migrate
you can learn more via Laravel documentation
That’s all I hope I could help!