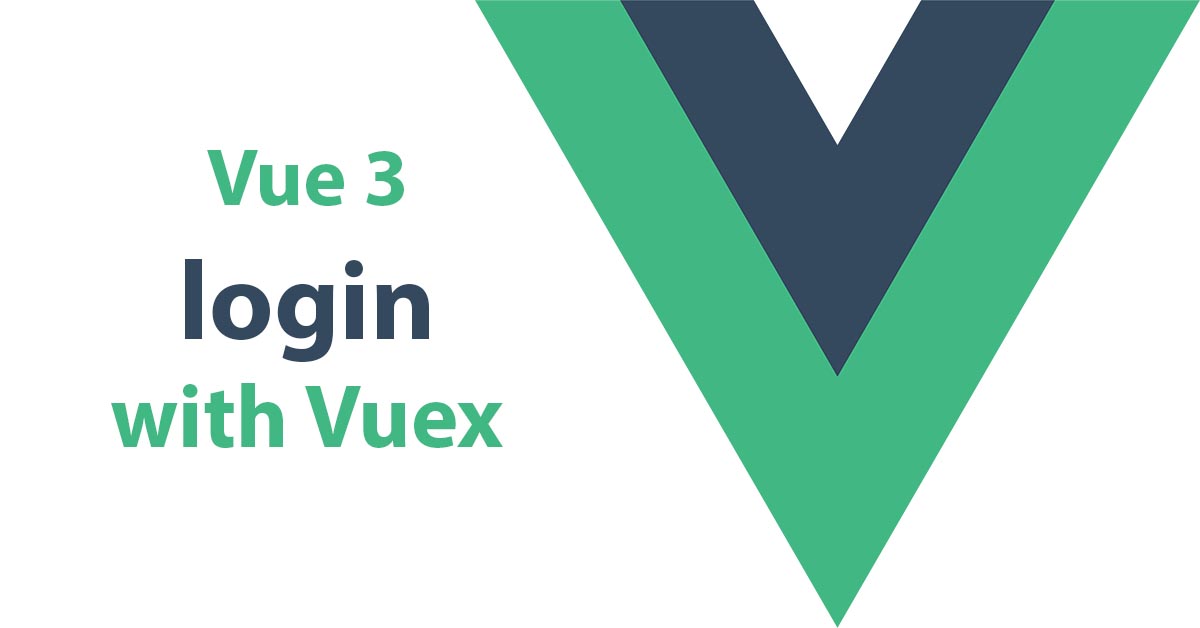
In this Vue 3 login example tutorial, we will cover using Vuex. And creating a store in a Vue.js app for making a user login.
Vue.js is a good choice for creating a single-page website. In fact, it has everything we need to start development. A package such as Vuex is also a good choice for creating sessions for a logged-in user. Also, Vuex is The official state management library for Vue. That means you can save states for your application and call them wherever you want as long as the user uses the website but if the user closes it everything is lost. But, thanks to today’s browsers that have web storage API that allow us to save our session data on the users’ browser for the next time they open our website.
For creating Vue 3 login example, We will use Vue CLI , I have created a tutorial before How to Create Vuejs Website with Vue CLI And Vue Router. But in this tutorial we are digging deeper with Vue 3.
Login steps
When the user open the website Vuex will check if it have saved an email before, If no, the user can log in, if yes, the user can log out.
Creating Vue 3 website with Vue CLI
I suppose you have Vue CLI and Node.js up and running on your systmen.So Let’s create our website.
vue create login-app
When it asks “Please pick a preset?” select Vue 3
After, it finishes go to the project folder and run the server as following
cd login-app
npm run serve
Then click the link in the terminal “http://localhost:8080/
” to make sure everything is alright.
After that, let’s install Vue-router for creating routes and pages.
vue add router
We will use history mode for router. So enter Y
when it asks.
Setting up Vuex
To install vuex in our website run the following code.
vue add vuex
It will create a new folder named “store
” and “index.js
” file in “src” directory. And all we care about is state
and mutations
.
State
is a vuex object, we use it for store the appication states. For example saving the user email, name, access token.
mutations
you can think of it as an event. Because The only way to change state in a Vuex store by committing a mutation.
You can learn more about Vuex objects on its official wbsite.
import { createStore } from 'vuex'
export default createStore({
state: {
email:""
},
getters: {
},
mutations: {
login (state,email) {
state.email = email;
localStorage.setItem('email', email);
alert('Logged in');
},
logout(state){
state.email ="";
localStorage.removeItem('email');
alert('Logged out');
},
initialiseStore(state) {
if(localStorage.getItem('email')){
state.email = localStorage.getItem('email');
}
}
},
actions: {
},
modules: {
}
})
- We added
email
tostate
. Because that will be the user’s email and for checking if the user logged in or not. - In
mutations
we created thelogin
, andlogout
function and we used thelocalStrorage
API for saving the email in the browser local storage. - We use
initialiseStore
to check if the user logged in or not.
Creating the login page
To create the login page, first, we need to add the route. So open src\router\index.js
file and add its route as the following
import { createRouter, createWebHistory } from 'vue-router'
import HomeView from '../views/HomeView.vue'
const routes = [
{
path: '/',
name: 'home',
component: HomeView
},
{
path: '/about',
name: 'about',
// route level code-splitting
// this generates a separate chunk (about.[hash].js) for this route
// which is lazy-loaded when the route is visited.
component: () => import(/* webpackChunkName: "about" */ '../views/AboutView.vue')
},
//login page
{
path: '/login',
name: 'login',
component: () => import(/* webpackChunkName: "about" */ '../views/LoginView.vue')
}
]
const router = createRouter({
history: createWebHistory(process.env.BASE_URL),
routes
})
export default router
Create a new view component src\views\LoginView.vue
<template>
<form @submit="Submit" method="post">
<label for="uname"><b>Email</b></label>
<input type="email" placeholder="Enter Email" v-model="form.email" required>
<br><br>
<label for="psw"><b>Password</b></label>
<input type="password" placeholder="Enter Password" v-model="form.password" required>
<br><br>
<button type="submit">Login</button>
</form>
<br>
{{$store.state.email}}
</template>
<script>
export default {
data() {
return {
form: {
email:"",
password:""
}
}
},
methods :{
Submit(event){
event.preventDefault()
this.$store.commit('login',this.form.email)
}
}
}
</script>
- We created the login form to be submitted by the submit method.
-
$store.state.email
this is how to use the vuex store state inside a component template. And if you want to use it inside a script, Just like thatthis.$store.state.email
. - To commit any
mutations function
, we do thatthis.$store.commit('function-name','parameter')
. That’s what we do in theSubmit
methodthis.$store.commit('login',this.form.email)
.
Check if the user logged in
It’s so easy to do, in src\App.vue
we will add these script. so when the user open the website, it will run the vuex initialiseStore
.
<script>
export default {
mounted(){
this.$store.commit('initialiseStore')
}
}
</script>
Adding Vuex Logout
To make the user able to logout from your website we will add this method in src\App.vue
methods:{
logout(){
this.$store.commit('logout')
}
},
In the template
add the following change for toggling between login and logout links at the navigation bar.
<template>
<nav>
<router-link to="/">Home</router-link> |
<router-link to="/about">About</router-link>|
<router-link v-if="!$store.state.email" to="/login">Login</router-link>
<a v-if="$store.state.email" @click="logout">Logout</a>
</nav>
<router-view/>
</template>
Conclusion
We save the application state in Vuex and if we want to keep the data on the user’s browser for the next time, we use localStorage
. You should use server side calls to secure your application too. Such as access tokens and permissions. All sensetive data should be saved on the backend.